What makes ZeptoMail special?
Mailgun is an email service for developers that allows them to send, receive, and monitor emails. It can be used to send both transactional emails and marketing emails. Mailgun offers different features, but it requires an expensive upgrade to use most of the important features.
If your primary focus is to send transactional emails, why would you pay for two different services?
With Zoho ZeptoMail, you pay for what you use. ZeptoMail is a dedicated transactional email platform used to send transactional emails ensuring email delivery speed and inbox placement. Unlike Mailgun, you get to use all of the features regardless of what you pay.
Get started with Zoho ZeptoMail.
Make the move from Mailgun to Zoho ZeptoMail following this simple four-step guide.
Sign up for Zoho ZeptoMail.
Sign up for Zoho ZeptoMail by providing the necessary credentials.
You’ll get a verification email to confirm your email address.
Once your account is verified, you’ll need to set up two-factor authentication by selecting from the available options.
Add and verify your domain.
After you verify your account, add your domain address and bounce address.
Configure DKIM and CNAME records in your DNS configuration to verify your domain.
Refer to this step-by-step guide on how to add and verify your domain.
Modify one line of your existing code.
You can transition from Mailgun to ZeptoMail by changing just one line of your API code.
Make an API call to https://api.zeptomail.com/v1.1/mg/email
To authenticate the request, include the send mail token in the header.
Welcome aboard!
Welcome to ZeptoMail. You’re all set to send your transactional emails from ZeptoMail. Happy email sending :)

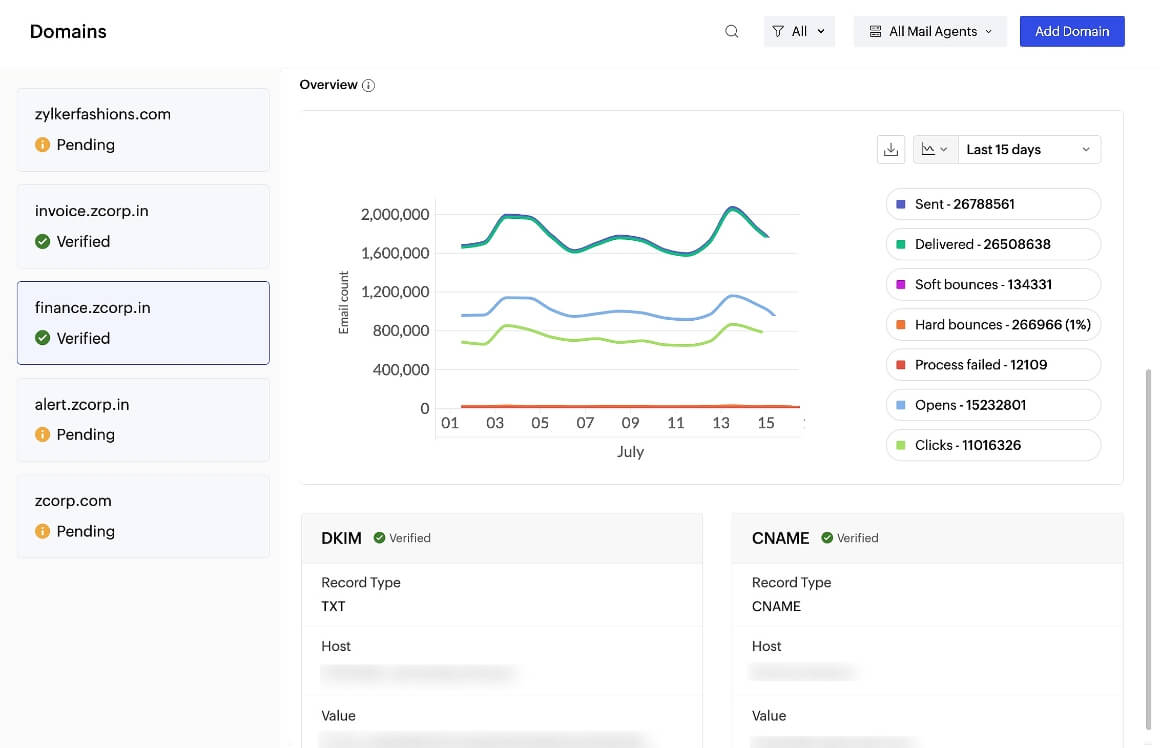
curl -X POST "https://api.zeptomail.com/v1.1/mg/email" \
-H "Authorization: Zoho-enczapikey YOUR_API_KEY_HERE" \
-H "Content-Type: application/x-www-form-urlencoded" \
--data "from=sender@example.in" \
--data "to=recipient1@example.com,recipient2@example.com" \
--data "subject=Test Email" \
--data "text=This is a test email." \
--data "html=Test Email" \
--data "cc=recipient3@example.com,recipient4@example.com" \
--data "bcc=recipient5@example.com,recipient6@example.com"
let formdata = new FormData();
formdata.append("from", "sender@example.com");
formdata.append("to", "recipient1@example.com,recipient2@example.com");
formdata.append("subject", "Test Email");
formdata.append("text", "Hello, this is test Email");
formdata.append("html", "<div>Hello, this is test Email</div>");
formdata.append("cc", "cc1@example.com,cc2@example.com");
formdata.append("bcc", "bcc1@example.com,bcc2@example.com");
fetch("https://api.zeptomail.com/v1.1/mg/email", {
body: formdata,
method: "POST",
headers: {
"Authorization": "Zoho-enczapikey [your-api-key]",
}
})
.then(async (resp) => {
let result = await resp.json();
console.log(result,result.details);
})
.catch((err) => {
console.log(err);
});
using System;
using System.Collections.Specialized;
using System.Net;
using System.Text;
public class Program
{
public static void Main()
{
string url = "https://api.zeptomail.com/v1.1/mg/email";
var formData = new NameValueCollection
{
{ "from", "sender@example.com" },
{ "to", "recipient1@example.com","recipient2@example.com" },
{ "subject", "Test Email" },
{ "text", "This is a test email." },
{ "html", "<div>>b>Test Email>/b>>/div>" },
{ "cc", "recipient3@example.com,recipient4@example.com" },
{ "bcc", "recipient5@example.com,recipient6@example.com" }
};
using (var client = new WebClient())
{
client.Headers.Add("Authorization", "Zoho-enczapikey [your-api-key]");
try
{
byte[] responseBytes = client.UploadValues(url, "POST", formData);
string responseBody = Encoding.UTF8.GetString(responseBytes);
Console.WriteLine(responseBody);
}
catch (WebException e)
{
Console.WriteLine("Error: " + e.Message);
if (e.Response != null)
{
using (var reader = new System.IO.StreamReader(e.Response.GetResponseStream()))
{
Console.WriteLine(reader.ReadToEnd());
}
}
}
}
}
}
import requests
from requests_toolbelt.multipart.encoder import MultipartEncoder
# Creating form data for the request
formdata = MultipartEncoder(
fields={
"from": "sender@example.in",
"to": "recipient1@example.com,recipient2@example.com",
"subject": "Test Email",
"text": "This is a test email.",
"html": "<div><b>Test Email</b></div>",
"cc": "recipient3@example.com,recipient4@example.com",
"bcc": "recipient5@example.com,recipient6@example.com"
}
)
# API endpoint URL
url = "https://api.zeptomail.com/v1.1/mg/email"
# Headers including authorization and content type
headers = {
"Authorization": "Zoho-enczapikey YOUR_API_KEY_HERE",
"Content-Type": formdata.content_type # Required for multipart form data
}
# Sending POST request to the API endpoint
try:
response = requests.post(url, headers=headers, data=formdata)
response.raise_for_status() # Raise error if HTTP request failed
result = response.json() # Parse JSON response
print(result)
except requests.exceptions.RequestException as err:
print(f"Error: {err}")
<?php
// API endpoint URL
$url = "https://api.zeptomail.com/v1.1/mg/email";
// Form data as an array
$formData = [
"from" => "sender@example.in",
"to" => "recipient1@example.com,recipient2@example.com",
"subject" => "Test Email",
"text" => "This is a test email.",
"html" => "<div><b>Test Email</b> </div>",
"cc" => "recipient3@example.com,recipient4@example.com",
"bcc" => "recipient5@example.com,recipient6@example.com"
];
// Authorization header
$headers = [
"Authorization: Zoho-enczapikey YOUR_API_KEY_HERE"
];
// Initialize cURL session
$ch = curl_init($url);
// cURL options
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
curl_setopt($ch, CURLOPT_POSTFIELDS, $formData);
// Execute the POST request
$response = curl_exec($ch);
// Check for errors in the request
if ($response === false) {
$error = curl_error($ch);
echo "Error: $error";
} else {
// Parse and print the response
$result = json_decode($response, true);
print_r($result);
}
// Close cURL session
curl_close($ch);
?>
import org.apache.http.HttpEntity;
import org.apache.http.HttpResponse;
import org.apache.http.client.methods.CloseableHttpResponse;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.entity.ContentType;
import org.apache.http.entity.mime.MultipartEntityBuilder;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClients;
import org.apache.http.util.EntityUtils;
public class ZeptoMailMultipart {
public static void main(String[] args) {
String url = "https://api.zeptomail.com/v1.1/mg/email";
String apiKey = "Zoho-enczapikey YOUR_API_KEY_HERE";
// Build the multipart form data
MultipartEntityBuilder builder = MultipartEntityBuilder.create();
builder.addTextBody("from", "sender@example.in", ContentType.TEXT_PLAIN);
builder.addTextBody("to", "recipient1@example.com,recipient2@example.com", ContentType.TEXT_PLAIN);
builder.addTextBody("subject", "Test Email", ContentType.TEXT_PLAIN);
builder.addTextBody("text", "This is a test email.", ContentType.TEXT_PLAIN);
builder.addTextBody("html", "<div><b>Test Email</b> </div>", ContentType.TEXT_HTML);
builder.addTextBody("cc", "recipient3@example.com,recipient4@example.com", ContentType.TEXT_PLAIN);
builder.addTextBody("bcc", "recipient5@example.com,recipient6@example.com", ContentType.TEXT_PLAIN);
HttpEntity multipart = builder.build();
// Send the POST request
try (CloseableHttpClient client = HttpClients.createDefault()) {
HttpPost post = new HttpPost(url);
post.setHeader("Authorization", apiKey);
post.setEntity(multipart);
CloseableHttpResponse response = client.execute(post);
HttpEntity responseEntity = response.getEntity();
String result = EntityUtils.toString(responseEntity);
System.out.println("Response: " + result);
} catch (Exception e) {
e.printStackTrace();
}
}
}

How is ZeptoMail different from Mailgun?
The concepts and terminologies will help you navigate Zoho ZeptoMail.
- Email segments
- DNS Requirements
- Attachment Management
- User Management
- User Activity Logs
- Measure
- Suppress Emails
- Email Logs
- Email Trackers
- Logs and Content Retention
- Subscription
- Support
- IPs
- Webhooks
- Domain Settings → DNS recordsEnter your SPF, DKIM, MX, and CNAME under DNS records to authenticate your domain.
- Account usersManage ownership of multi-users in an account.(Only applicable to upgraded users)
- User sessionsThe app records all of the user’s activity in user-sessions.
- AnalyticsReceive reports of accepted, delivered, opened, and failed mails.Upgrade your account to add a custom domain and view in-depth analytics about the messages you send.
- SuppressionsBounces, complaints, unsubscribes, and whitelists are added to suppression lists manually or automatically.
- LogsLogs record the message events for every email address you reach.
- Enable trackingEnable click and open tracking for sending domains.
- Basic plan foundation5 days of log retention. 1 day of message retention.(Even enterprise-level plans provide 30 days of log retention and 7 days of message retention).
- Scale for 100,000 emails$90 per month + additional charges for access to all features.Free for 30 days.
- 24/7 ticket support. You’ll need to upgrade for live phone support and instant chat support.
- IP addressesYou can add IP addresses associated to your account.
- Sending → webhooksWebhooks are added to domains to receive notifications on the activities linked to the messages you send.
- zeptomail
- Mail AgentsOrganize your emails using Mail Agents by type, purpose, app, and more.
- Domains → DNS settingsValidate your domain by configuring DKIM, and CNAME records within your DNS settings
- File CacheUpload attachments to the File Cache and save more space. You can insert the File Cache key when sending emails.The File Cache supports 1GB of storage for an account.
- Manage usersControl the ownership and permissions of multiple users on the account.(Applicable to all users)
- Activity logsActivity logs allow you to see the actions carried out by various users in your ZeptoMail account.
- ReportsGet detailed insights into sent, delivered, soft bounces, hard bounces, process failed, opens, clicks, and custom reports for all users.
- Suppression listAutomatically, some hard bounces are added to the suppression list. You can also manually suppress email addresses and domains.
- Processed emailsProcessed emails are shown in the order they were sent.
- Email trackingTo track open and click events in ZeptoMail, you will have to activate tracking individually for each Mail Agent.
- Any number of credits60 days of processed email data retention and content retention (should be enabled in settings).1 year of activity log retention.
- 10 credits for 100,000 emails$25 (10 credits) for access to all features for all users.1 credit free (10,000 emails) for6 months.
- 24/7 technical support along with extensive phone and chat support.
- IP restrictionsAdd a list of IPs that will be used to send emails from ZeptoMail.
- Mail Agent → webhooksWebhooks should be configured to receive notifications about activities in your emails.(Webhooks are an optional feature for all users.)
Mailgun Transition API Code Explained
A detailed documentation into Mailgun transition API to start sending your transactional emails from ZeptoMail.
Mailgun Transition APIZeptoMail feature highlights
- Mail Agents
- File Cache
- Processed Emails
- Email Templates
- Webhooks
- Email Tracking
- Domains
- Suppression List
- Reports
- Subscription
- Manage Users
- Activity Logs
- Content Settings
- IP restrictions
- Export Logs
Why choose ZeptoMail?
Exclusively transactional
ZeptoMail offers a dedicated solution that guarantees quick delivery and inbox placement.
User-friendly interface
Integrate ZeptoMail into your business effortlessly with its easy-to-use interface.
Email segmentation
Sort your emails into Mail Agents according to domain, application, purpose, and other criteria.
No gatekeeping
Enjoy uninterrupted access to all features. ZeptoMail's functionalities require no updates or additional expenses.
Comprehensive reports
ZeptoMail's detailed reports and logs keep you updated on all of your activities, documenting each action as data for complete tracking.
Flexible pricing
Say goodbye to monthly plan payments and unused email costs with ZeptoMail's pay-as-you-go pricing model.
Attachment storage
Use ZeptoMail's File Cache feature to save and upload attachments for smoother email sending.
24/7 support
Connect with ZeptoMail's chat, phone, and email support for any assistance related to ZeptoMail.
Security and privacy
ZeptoMail prioritizes security, taking a "security-first" stance given the sensitive nature of transactional email content.
Frequently asked questions
Switch to experience secure email sending at an affordable pricing.
All names and marks mentioned here remain the property of their original owners. Details are as published by the named competitors on their website(s) on April 2024 and are subject to change without notice. The details provided on this page are for general purposes only and cannot be considered as authorized information from the respective competitors. Zoho disclaims any liability for possible errors, omissions, or consequential losses based on the details here.