What makes Zoho ZeptoMail special?
Amazon Simple Email Service (Amazon SES) offers competitive pricing but requires additional payment for some basic features. Businesses seeking detailed tracking and 24/7 support for transactional emails may find Amazon SES less intuitive.
Why spend time configuring features when they should be easily accessible?
ZeptoMail, a specialized transactional email service, prioritizes fast and secure delivery. Unlike Amazon SES, all of our features are accessible with a single click, without complex setups or addons. Additionally, we offer 24/7 technical support at no extra cost.
Get started with Zoho ZeptoMail.
Follow this four-step guide for a smooth transition from Amazon SES to Zoho ZeptoMail.
Sign up for Zoho ZeptoMail.
Sign up for Zoho ZeptoMail by entering the relevant credentials.
Once you sign up, you’ll receive a verification email to validate your email address.
After you verify your account, you’ll be asked to enable two-factor authentication (TFA) for your account. You can choose from the list of TFA options to enable it.
Domain addition and verification
As soon as you complete your account verification, you can start adding your domain address.
Set up DKIM, and CNAME records in your DNS configuration to verify your domain.
Learn more from this step-by-step guide on how to add and verify your domain.
Modify one line of your existing code
You can easily switch from Amazon SES to ZeptoMail by just changing this one line of your API code.
Send an API request to https://api.zeptomail.com/v1.1/as/email
To authorize your request, you need to add the Send mail token in the header section.
Send transactional emails from ZeptoMail
Welcome to Zoho ZeptoMail. You can start sending your transactional emails via ZeptoMail. Happy email sending!

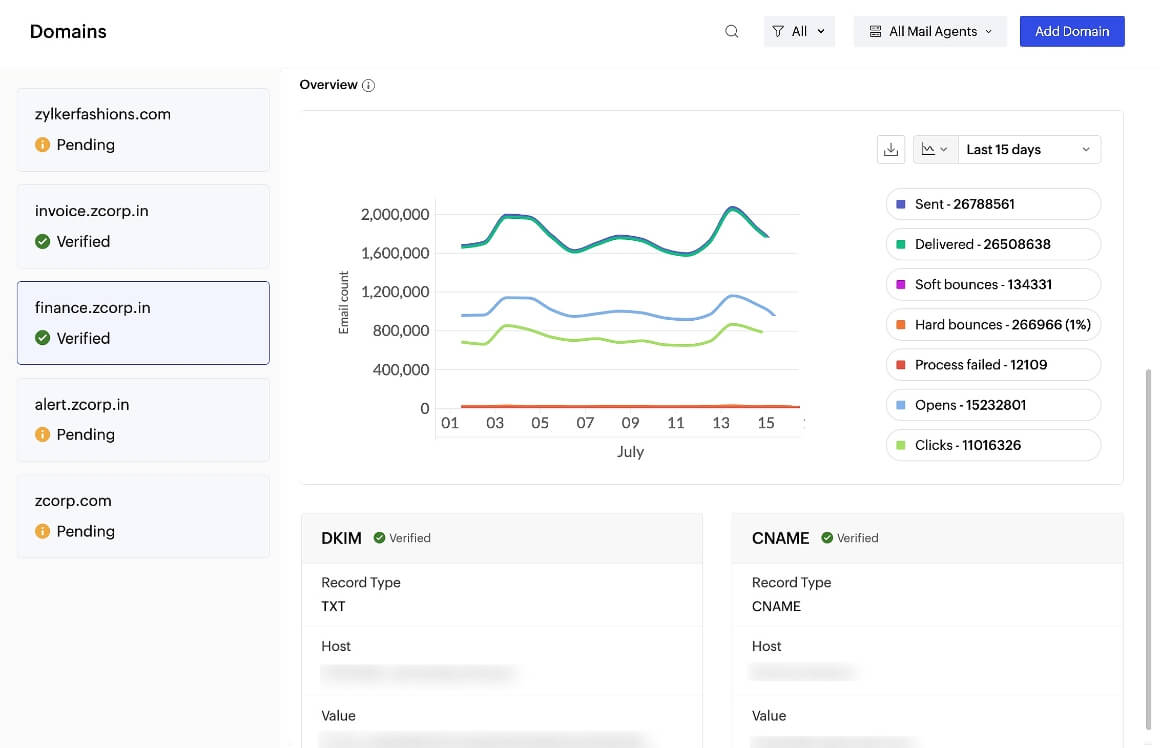
curl -X POST https://api.zeptomail.com/v1.1/as/email \
-H "Authorization: Zoho-enczapikey YOUR_API_KEY_HERE" \
-H "Accept: application/json" \
-H "Content-Type: application/json" \
-d '{
"Content": {
"Simple": {
"Body": {
"Html": {
"Charset": "UTF-8",
"Data": "<p>Hello</p>"
},
"Text": {
"Charset": "UTF-8",
"Data": "Hello"
}
},
"Subject": {
"Charset": "UTF-8",
"Data": "Test subject"
}
}
},
"Destination": {
"BccAddresses": [
"recipient1@example.com"
],
"CcAddresses": [
"recipient2@example.com"
],
"ToAddresses": [
"recipient3@example.com"
]
},
"FeedbackForwardingEmailAddress": "bounce@example.com",
"FromEmailAddress": "sender@example.com",
"ReplyToAddresses": [
"reply@example.com"
]
}'
fetch("https://api.zeptomail.com/v1.1/as/email", {
body: JSON.stringify({
"Content": {
"Simple": {
"Body": {
"Html": {
"Charset": " UTF-8",
"Data": "<p>Hello</p>"
},
"Text": {
"Charset": "UTF-8",
"Data": "Hello"
}
},
"Subject": {
"Charset": "UTF-8",
"Data": "Test subject"
}
}
},
"Destination": {
"BccAddresses": [
"bcc@example.com"
],
"CcAddresses": [
"cc@example.com"
],
"ToAddresses": [
"recipient@example.com"
]
},
"FeedbackForwardingEmailAddress": "bounce@example.com",
"FromEmailAddress": "sender@example.com",
"ReplyToAddresses": [
"reply@example.com"
]
}),
method: "POST",
headers: {
"Authorization": "Zoho-enczapikey [your-api-key]",
"Accept": "application/json",
"Content-Type": "application/json"
}
})
.then(async (resp) => {
if (resp.ok) {
let result = await resp.json();
console.log(result);
} else {
console.log(`Error: ${resp.statusText}`);
const errorBody = await resp.text();
console.log(`Error body: ${errorBody}`);
}
})
.catch((err) => {
console.log('Error sending email:', err);
});
using System;
using System.IO;
using System.Net;
using System.Text;
using Newtonsoft.Json;
namespace ZeptoMailRequest
{
public class Program
{
public static void Main(string[] args)
{
ServicePointManager.SecurityProtocol = SecurityProtocolType.Tls12;
var baseAddress = "https://api.zeptomail.com/v1.1/as/email";
var http = (HttpWebRequest)WebRequest.Create(new Uri(baseAddress));
http.Accept = "application/json";
http.ContentType = "application/json";
http.Method = "POST";
http.PreAuthenticate = true;
http.Headers.Add("Authorization", "Zoho-enczapikey [your-api-key]");
var payload = new
{
Content = new
{
Simple = new
{
Body = new
{
Html = new
{
Charset = "UTF-8",
Data = "<p>Hello</p>"
},
Text = new
{
Charset = "UTF-8",
Data = "Hello"
}
},
Subject = new
{
Charset = "UTF-8",
Data = "Test subject"
}
}
},
Destination = new
{
BccAddresses = new[] { "recipient1@example.com" },
CcAddresses = new[] { "recipient2@example.com" },
ToAddresses = new[] { "recipient3@example.com" }
},
FeedbackForwardingEmailAddress = "bounce@example.com",
FromEmailAddress = "from@example.com",
ReplyToAddresses = new[] { "reply@example.com" }
};
string jsonPayload = JsonConvert.SerializeObject(payload);
byte[] bytes = Encoding.UTF8.GetBytes(jsonPayload);
using (Stream newStream = http.GetRequestStream())
{
newStream.Write(bytes, 0, bytes.Length);
}
using (var response = http.GetResponse())
{
using (var stream = response.GetResponseStream())
{
using (var sr = new StreamReader(stream))
{
var content = sr.ReadToEnd();
Console.WriteLine(content);
}
}
}
}
}
}
import requests
import json
# API endpoint URL
url = "https://api.zeptomail.com/v1.1/as/email"
# Payload containing email details
payload = {
"Content": {
"Simple": {
"Body": {
"Html": {
"Charset": "UTF-8",
"Data": "<p>Hello from Example</p>"
},
"Text": {
"Charset": "UTF-8",
"Data": "Hello from Example"
}
},
"Subject": {
"Charset": "UTF-8",
"Data": "Test subject"
}
}
},
"Destination": {
"BccAddresses": [
"recipient1@example.com"
],
"CcAddresses": [
"recipient2@example.com"
],
"ToAddresses": [
"recipient3@example.com"
]
},
"FeedbackForwardingEmailAddress": "bounce@example.com",
"FromEmailAddress": "sender@example.com",
"ReplyToAddresses": [
"reply@example.com"
]
}
# Headers containing authorization and content-type
headers = {
"Authorization": "Zoho-enczapikey YOUR_API_KEY_HERE",
"Accept": "application/json",
"Content-Type": "application/json"
}
# Sending POST request to the API endpoint
try:
response = requests.post(url, headers=headers, data=json.dumps(payload))
response.raise_for_status() # Raise error if HTTP request failed
result = response.json() # Parse JSON response
print(result)
except requests.exceptions.RequestException as err:
print(f"Error: {err}")
<?php
// API endpoint URL
$url = "https://api.zeptomail.com/v1.1/as/email";
// Payload containing email details
$payload = [
"Content" => [
"Simple" => [
"Body" => [
"Html" => [
"Charset" => "UTF-8",
"Data" => "<p>Hello from Example</p>"
],
"Text" => [
"Charset" => "UTF-8",
"Data" => "Hello from Example"
]
],
"Subject" => [
"Charset" => "UTF-8",
"Data" => "Test subject"
]
]
],
"Destination" => [
"BccAddresses" => [
"recipient1@example.com"
],
"CcAddresses" => [
"recipient2@example.com"
],
"ToAddresses" => [
"recipient3@example.com"
]
],
"FeedbackForwardingEmailAddress" => "bounce@example.com",
"FromEmailAddress" => "sender@example.com",
"ReplyToAddresses" => [
"reply@example.com"
]
];
// Headers containing authorization and content-type
$headers = [
"Authorization: Zoho-enczapikey YOUR_API_KEY_HERE",
"Accept: application/json",
"Content-Type: application/json"
];
// Initialize cURL session
$ch = curl_init($url);
// Set cURL options
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
curl_setopt($ch, CURLOPT_POSTFIELDS, json_encode($payload));
// Execute the POST request
$response = curl_exec($ch);
// Check for errors
if ($response === false) {
$error = curl_error($ch);
echo "Error: $error";
} else {
// Parse and print the response
$result = json_decode($response, true);
print_r($result);
}
// Close cURL session
curl_close($ch);
?>
import java.io.OutputStream;
import java.net.HttpURLConnection;
import java.net.URL;
import java.util.Scanner;
import org.json.JSONObject;
public class ZeptoMailApi {
public static void main(String[] args) {
try {
// API endpoint URL
URL url = new URL("https://api.zeptomail.com/v1.1/as/email");
// Create a JSON object for the payload
JSONObject payload = new JSONObject();
JSONObject htmlBody = new JSONObject();
htmlBody.put("Charset", "UTF-8");
htmlBody.put("Data", "<p>Hello</p>");
JSONObject textBody = new JSONObject();
textBody.put("Charset", "UTF-8");
textBody.put("Data", "Hello");
JSONObject body = new JSONObject();
body.put("Html", htmlBody);
body.put("Text", textBody);
JSONObject subject = new JSONObject();
subject.put("Charset", "UTF-8");
subject.put("Data", "Test subject");
JSONObject simple = new JSONObject();
simple.put("Body", body);
simple.put("Subject", subject);
JSONObject content = new JSONObject();
content.put("Simple", simple);
payload.put("Content", content);
JSONObject destination = new JSONObject();
destination.put("BccAddresses", new String[] {"recipient1@example.com"});
destination.put("CcAddresses", new String[] {"recipient2@example.com"});
destination.put("ToAddresses", new String[] {"recipient3@example.com"});
payload.put("Destination", destination);
payload.put("FeedbackForwardingEmailAddress", "bounce@example.com");
payload.put("FromEmailAddress", "sender@example.com");
payload.put("ReplyToAddresses", new String[] {"reply@example.com"});
// Set up the connection
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setRequestMethod("POST");
conn.setRequestProperty("Authorization", "Zoho-enczapikey YOUR_API_KEY_HERE");
conn.setRequestProperty("Accept", "application/json");
conn.setRequestProperty("Content-Type", "application/json");
conn.setDoOutput(true);
// Write payload to request body
try (OutputStream os = conn.getOutputStream()) {
byte[] input = payload.toString().getBytes("utf-8");
os.write(input, 0, input.length);
}
// Get the response
Scanner scanner = new Scanner(conn.getInputStream(), "UTF-8");
String response = scanner.useDelimiter("\\A").next();
scanner.close();
System.out.println("Response: " + response);
conn.disconnect();
} catch (Exception e) {
e.printStackTrace();
}
}
}

How is ZeptoMail different from Amazon SES?
Here are the concepts and terminologies that will help you easily navigate while using Zoho ZeptoMail.
- Email Segments
- DNS Requirements
- Attachment Management
- User Management
- User Activity Logs
- Measure
- Content Storage
- Activity Data Storage
- Suppress Emails
- Email Trackers
- Subscription
- Support
- IP Restrictions
- Webhooks
- Email Templates
- Configuration → Configuration SetsSegment your outbound messages by segregating into different subaccounts.
- Configuration → Identities → Create Identity → DomainUnder Domain, verify your domain by entering your SPF, DKIM, and CNAME details.
- Virtual Deliverability → Manager → Dashboard → Account StatisticsGet detailed statistics of sent, delivered, transient bounces, permanent bounces, complaints, clicks, and opens.
- You can store data in Amazon Kinesis. (This is a different service that needs to be purchased in order to store data).
- Configuration → Suppression ListsBounces and complaints are added to suppression lists manually and automatically.
- Settings → Additional Settings → Enable TrackingEnable engagement tracking to keep track of opens and clicks.
- For 50,000 emails$5.12: Pricing changes based on the features you pick. (This is basic pricing to send 50,000 emails).Extra to add attachments to your emails.
- 24/7 email, phone, and chat support is available for all users.
- Settings → WebhooksAdd webhooks to domains to receive notifications on events linked to the messages you send.
- Configuration → Email TemplatesEmail templates can be added only via API.
- zeptomail
- Mail AgentsMail Agents are used to sort your emails by type, purpose, application, and other criteria.
- Domains → DNS settingsValidate your domain through DKIM, and CNAME record configuration in your DNS settings.
- File CacheMaximize storage efficiency by uploading attachments to the File Cache. Use the File Cache key when sending emails through API.ZeptoMail’s File Cache feature supports 1GB of storage for an account.
- Manage usersRegulate the ownership and access control for multiple users under the account.(applicable to all users)
- Activity LogsBy accessing the activity logs, you can see the activities executed by different users within your ZeptoMail account.(1 year of activity log retention)
- ReportsObtain reports on sent, delivered, soft bounces, hard bounces, processing failures, opens, clicks, and custom reports for all users.
- Any number of creditsRetain content data for 60 days with ZeptoMail.
- Processed EmailsWithin the Processed Emails section, sent emails are displayed in the sequence they were processed.60 days of processed email data retention and content retention.
- Suppression ListHard bounces and unsubscribes are automatically or manually added to the suppression list.
- Mail Agent → Email TrackingOpen and click tracking for each Mail Agent to track the performance of emails in ZeptoMail.
- For 50,000 emails$12.50 (5 credits): Access all features for all users; valid for 6 months for every user.Buy credits and pay only for the used emails.1 credit free (10,000 emails) for 6 months.
- All users benefit from 24/7 extensive technical support via phone, email, and chat.
- IP RestrictionsAssign a list of IPs solely authorized for sending emails exclusively from your ZeptoMail account.
- Mail Agent → WebhooksEstablish webhooks to receive notifications about actions within your emails.
- Mail Agents → TemplatesBuilt-in email templates are available on ZeptoMail. You can also customize or create a new one.
Get a detailed comparison between Amazon SES and ZeptoMail.
Amazon SES vs. ZeptomailAmazon SES Transition API Code Explained
A detailed documentation into Amazon SES transition API to start sending your transactional emails from ZeptoMail.
Amazon SES Transition APIZeptoMail feature highlights
- Mail Agents
- File Cache
- Processed Emails
- Email Templates
- Webhooks
- Email Tracking
- Domains
- Suppression List
- Reports
- Subscription
- Manage Users
- Activity Logs
- Content Settings
- IP Restrictions
- Export Logs
Mail Agents
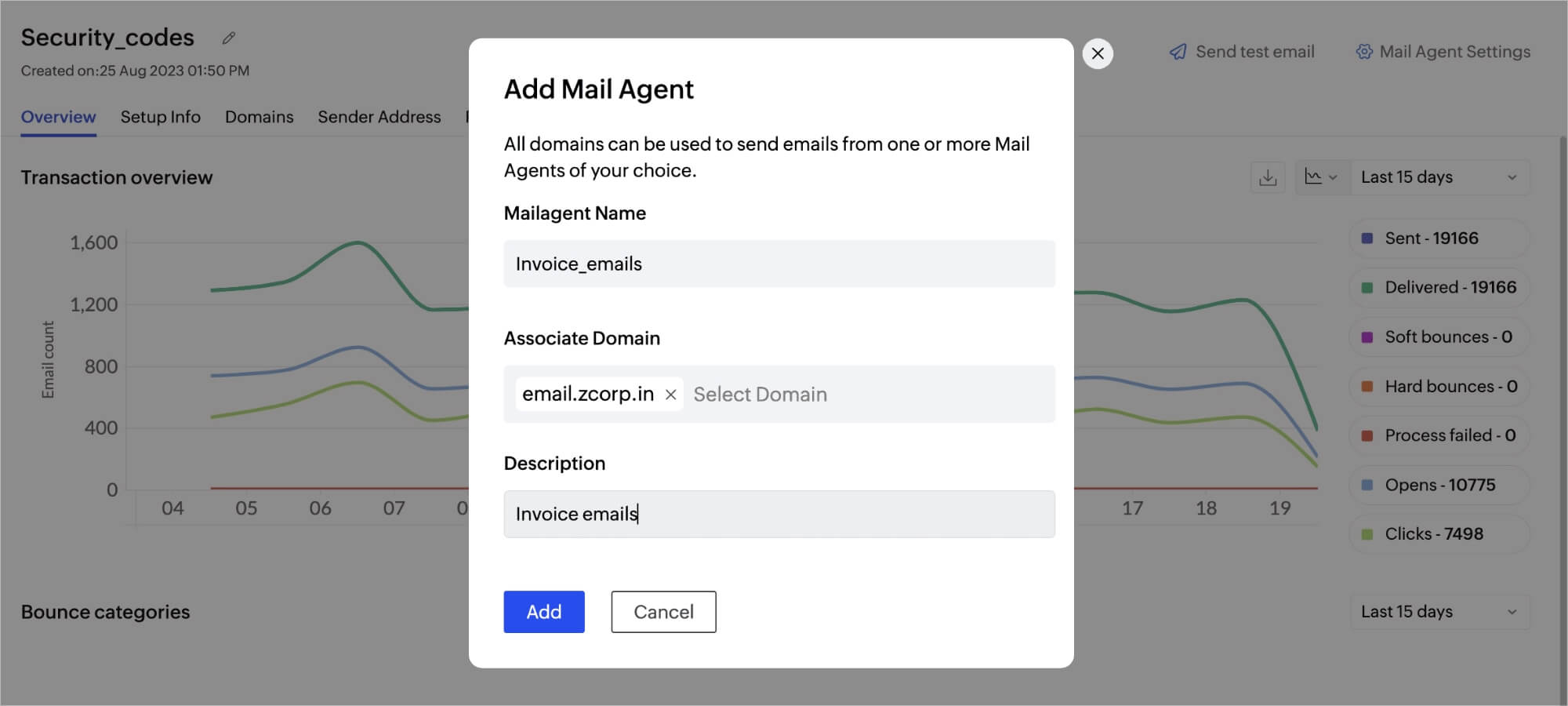
Mail Agent in ZeptoMail is designed to categorize your transactional emails based on their type, purpose, applications, and other criteria. This feature is beneficial for managing multiple applications or various transactional emails within your organization. Each Mail Agent is given a unique API token and SMTP credentials for authentication. Learn more
File Cache
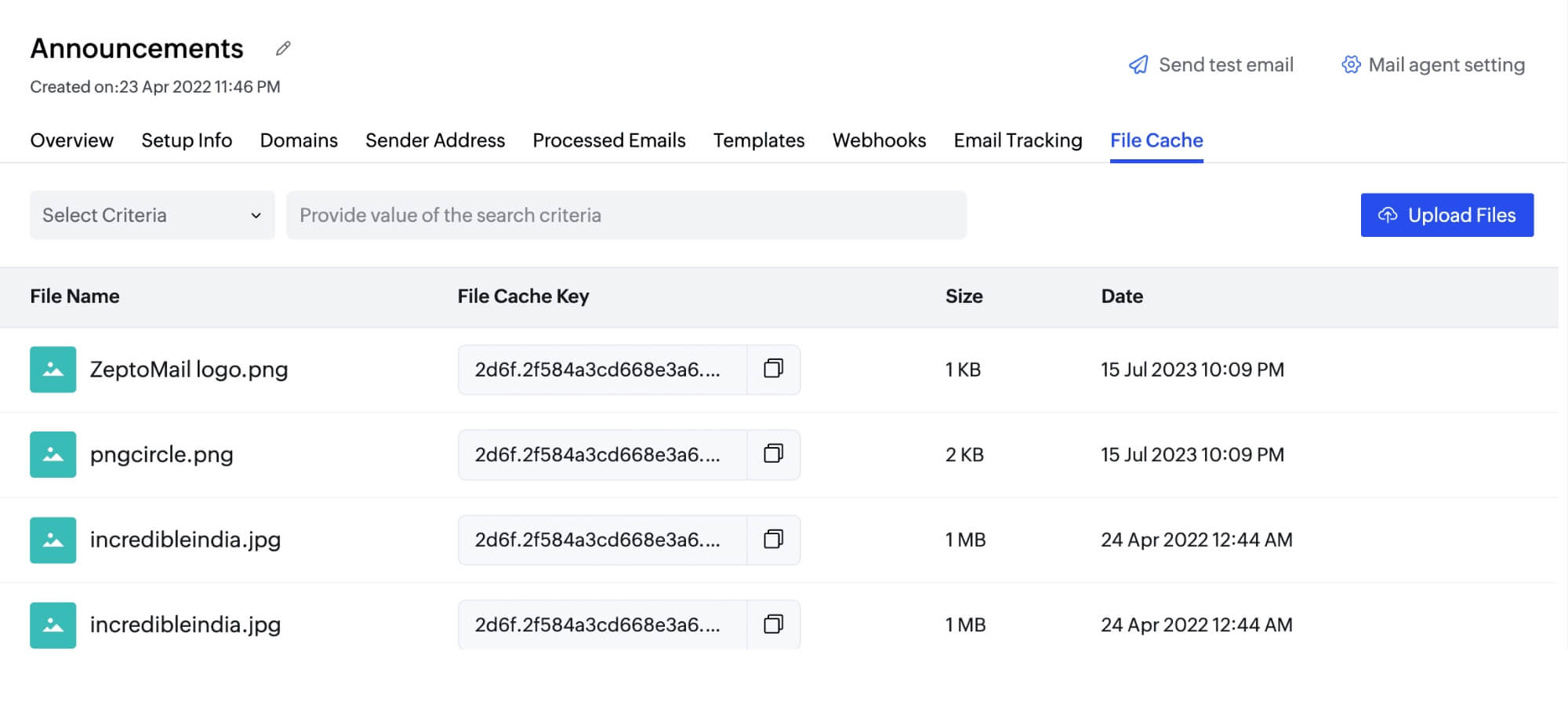
ZeptoMail introduces File Cache, a feature that simplifies attachment management by allowing you to add images to transactional emails via a File Cache key in the email API, removing the need for manual uploads. It offers up to 1GB of storage per account. Learn more
Processed Emails
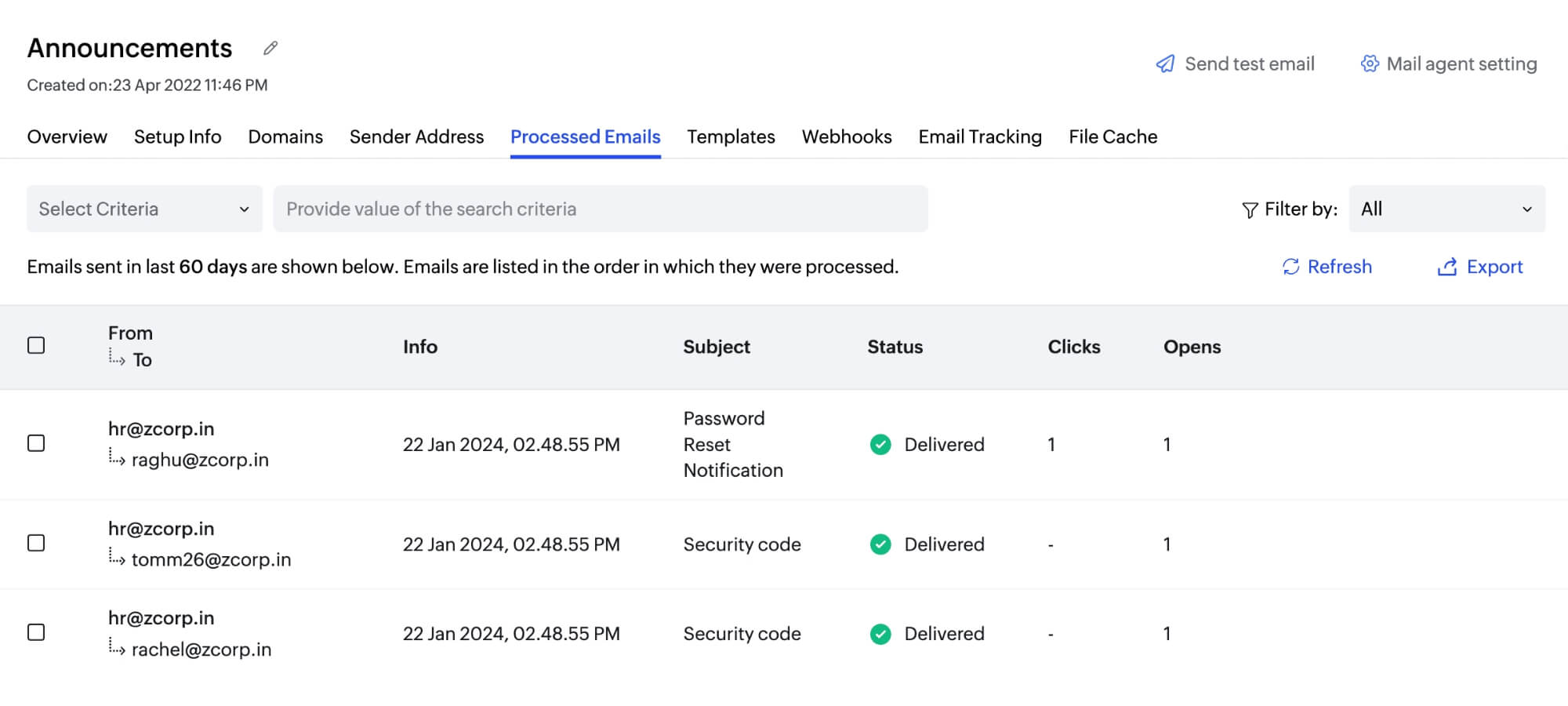
The Processed Emails feature provides visibility into the status of your sent emails, organized by their processing order. ZeptoMail stores this information for up to 60 days, with an option to export the list for tracking the performance of your transactional emails. Learn more
Email Templates
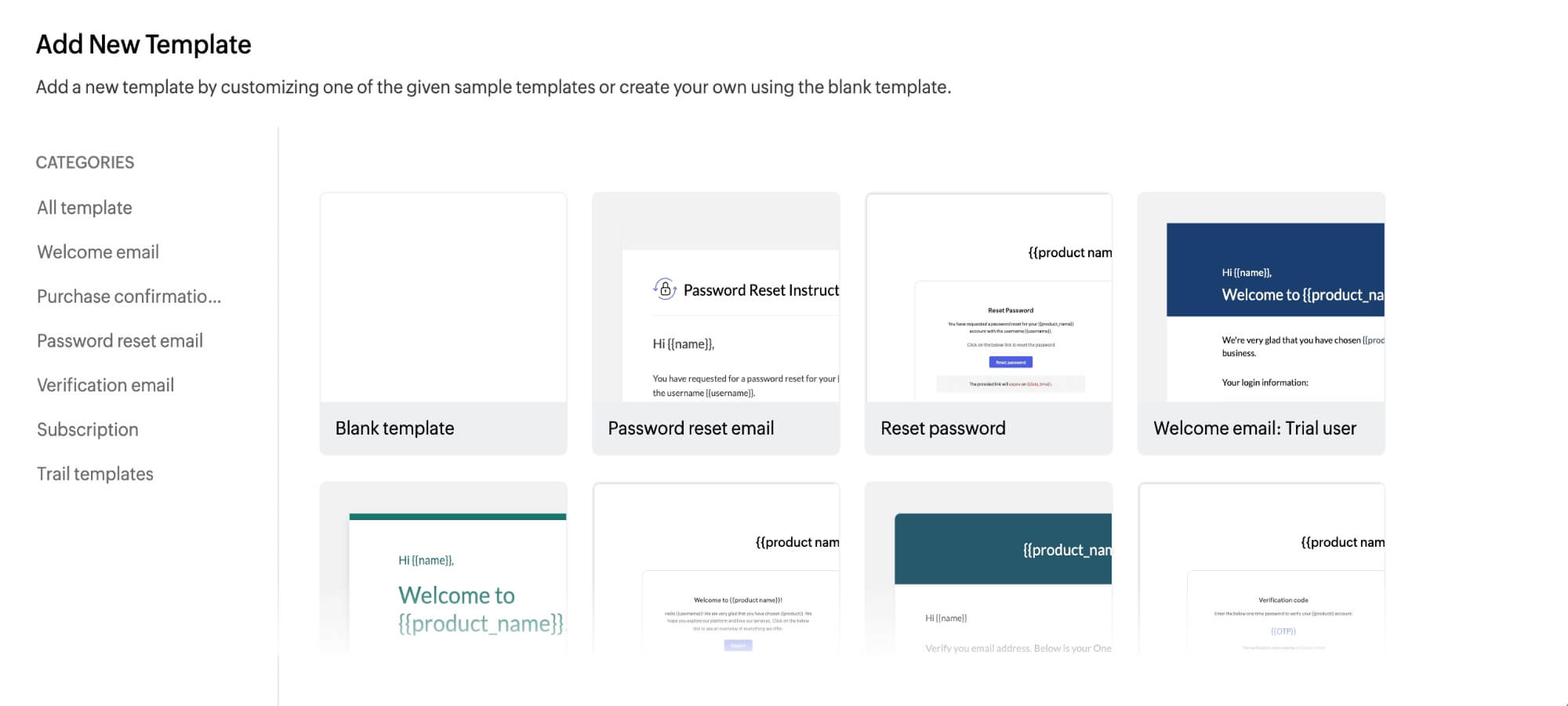
ZeptoMail provides ready-to-use transactional email templates, saving you from drafting the same email repeatedly. Whether it's OTPs, password resets, or order confirmations, you can use these templates to efficiently send emails to your users. Learn more
Webhooks
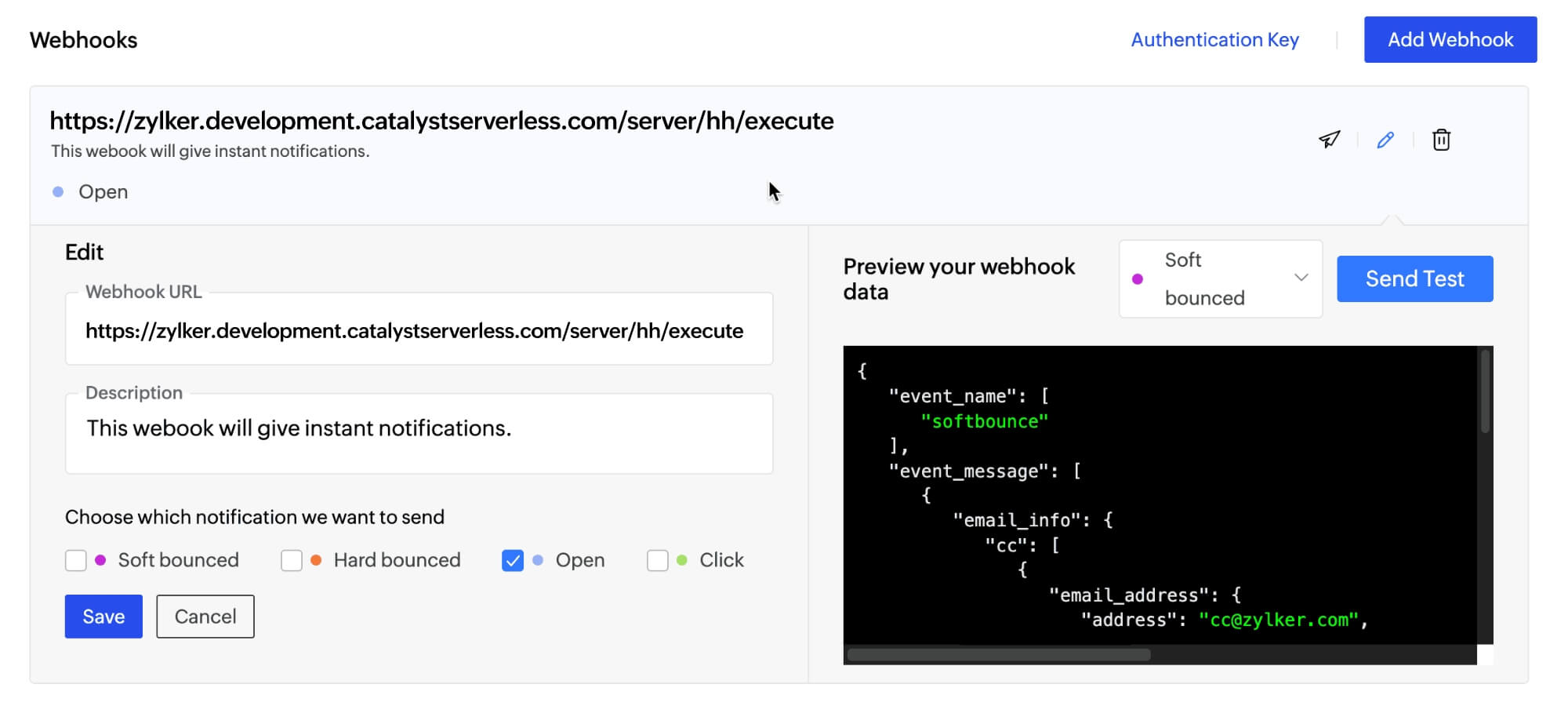
Webhooks are custom HTTP callbacks activated by specific events. In ZeptoMail, they instantly send recipient activity data (such as opens, clicks, and bounces) to your application as soon as these actions happen. When triggered, a webhook immediately sends data to the designated URL, offering real-time notifications. Learn more
Email Tracking
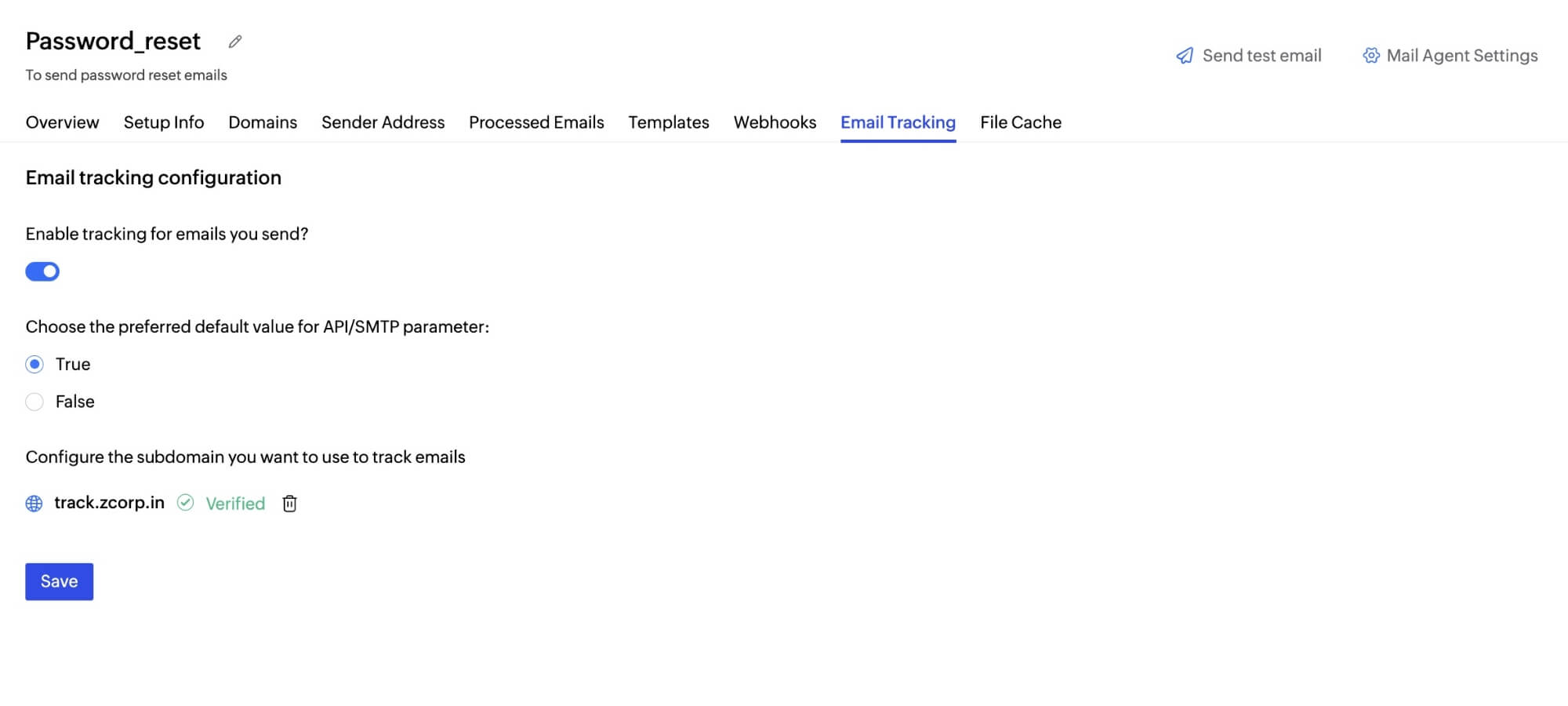
Email tracking allows you to monitor opens and clicks, giving you insight into the engagement levels of your transactional emails. In ZeptoMail, you must enable email tracking to keep track of these interactions. Remember to activate tracking individually for each Mail Agent. Learn more
Domains
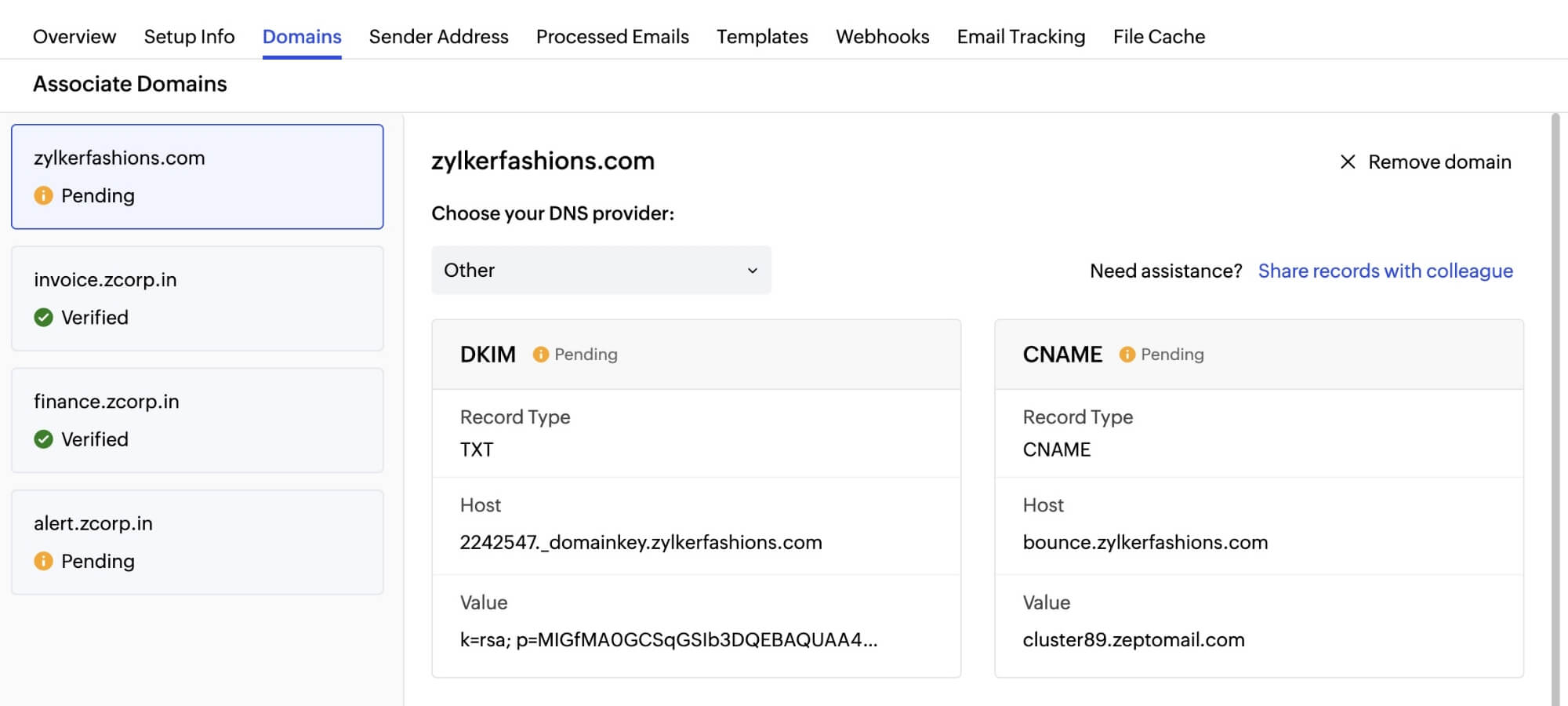
The Domains page lets you manage and add domains to ZeptoMail, with a maximum of 100 domains per account. On this page, you can access DKIM and CNAME values for DNS verification, view domains linked to each Mail Agent, and check their verification status. Learn more
Suppression List
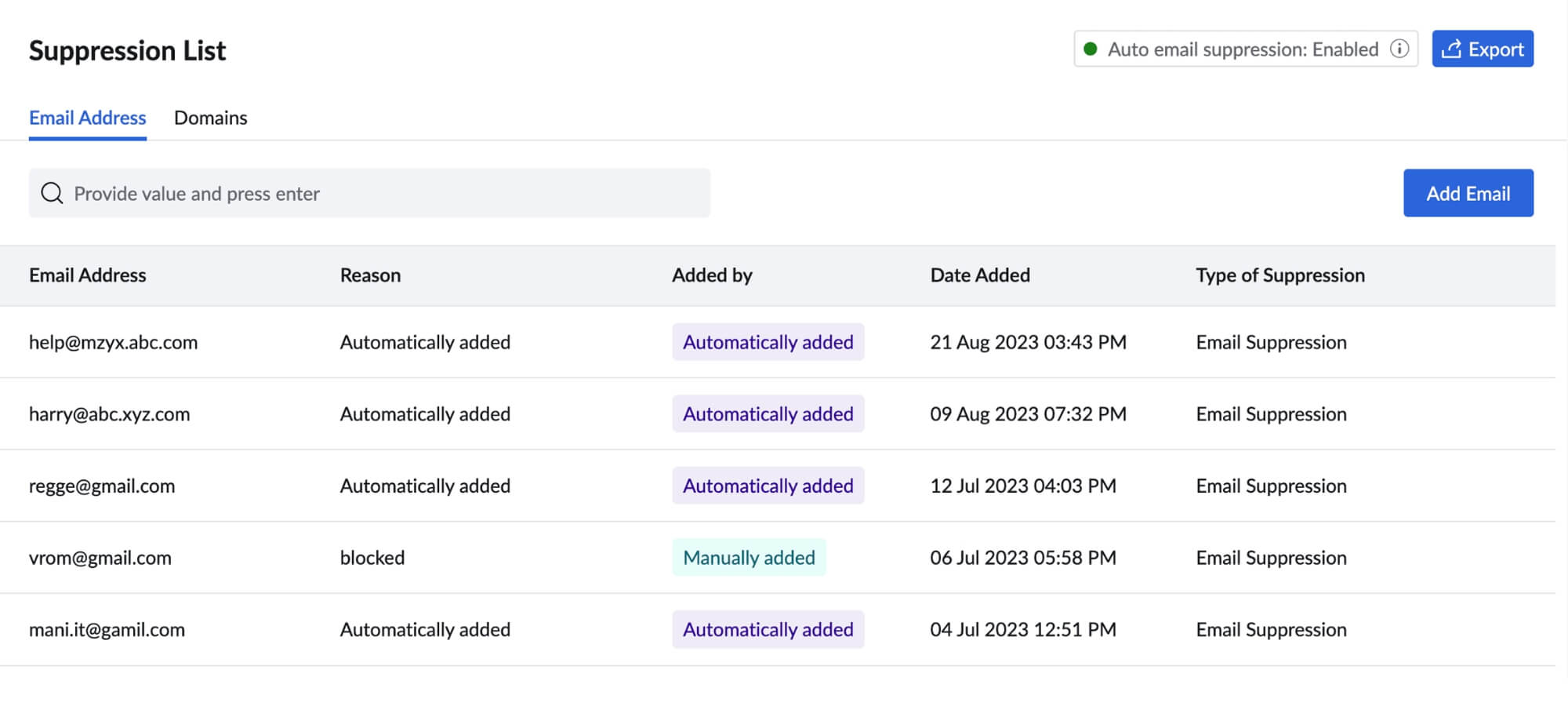
The Suppression List tracks email addresses and domains that are prevented from receiving emails due to issues like bounces, unsubscribes, or invalid addresses. With ZeptoMail's auto-suppression feature, bounced addresses can also be added to this list automatically. Learn more
Reports
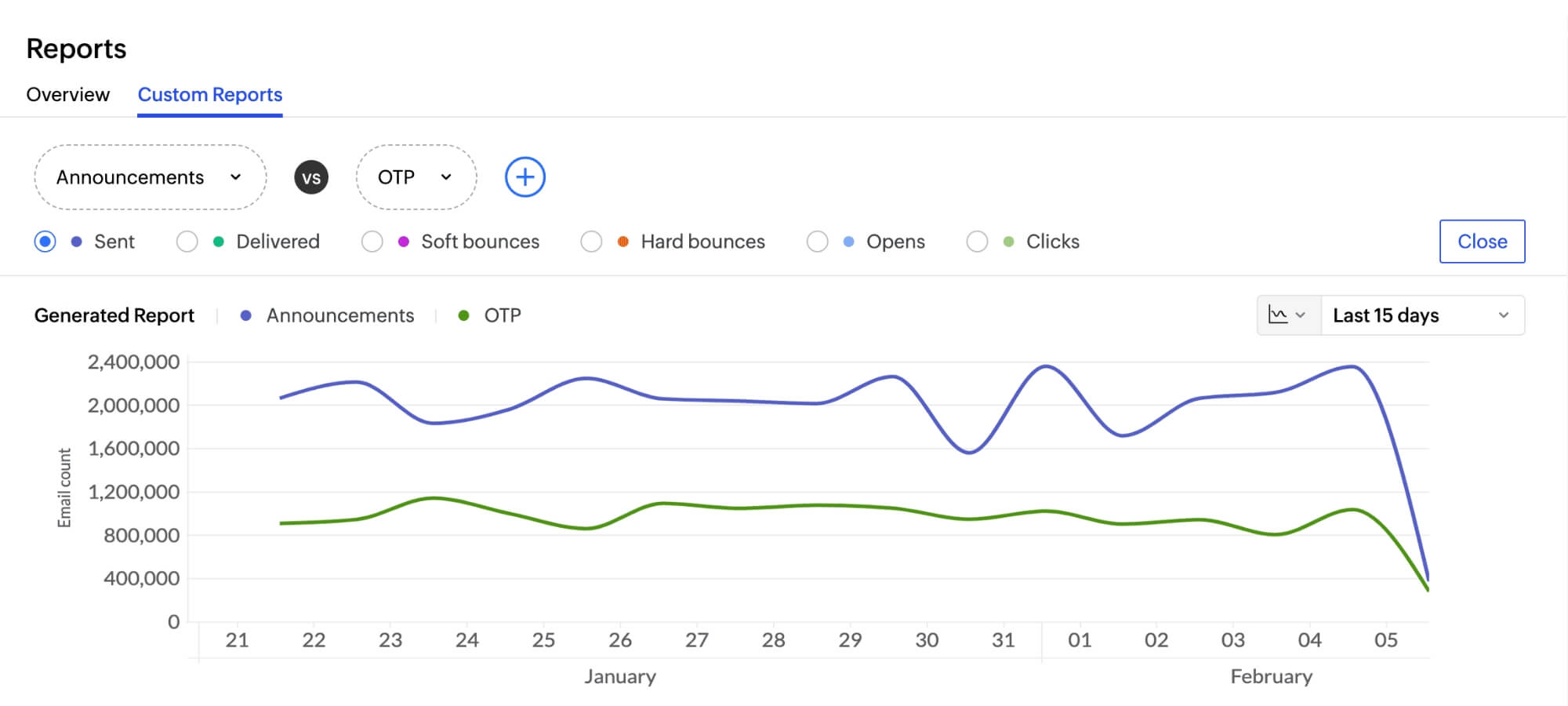
ZeptoMail lets you generate custom reports to track the performance of your transactional emails. You can effortlessly compare two Mail Agents and receive detailed reports on opens, bounces, clicks, user engagement, and more. Make sure to enable Email Tracking to gather data on clicks and opens. Learn more
Subscription
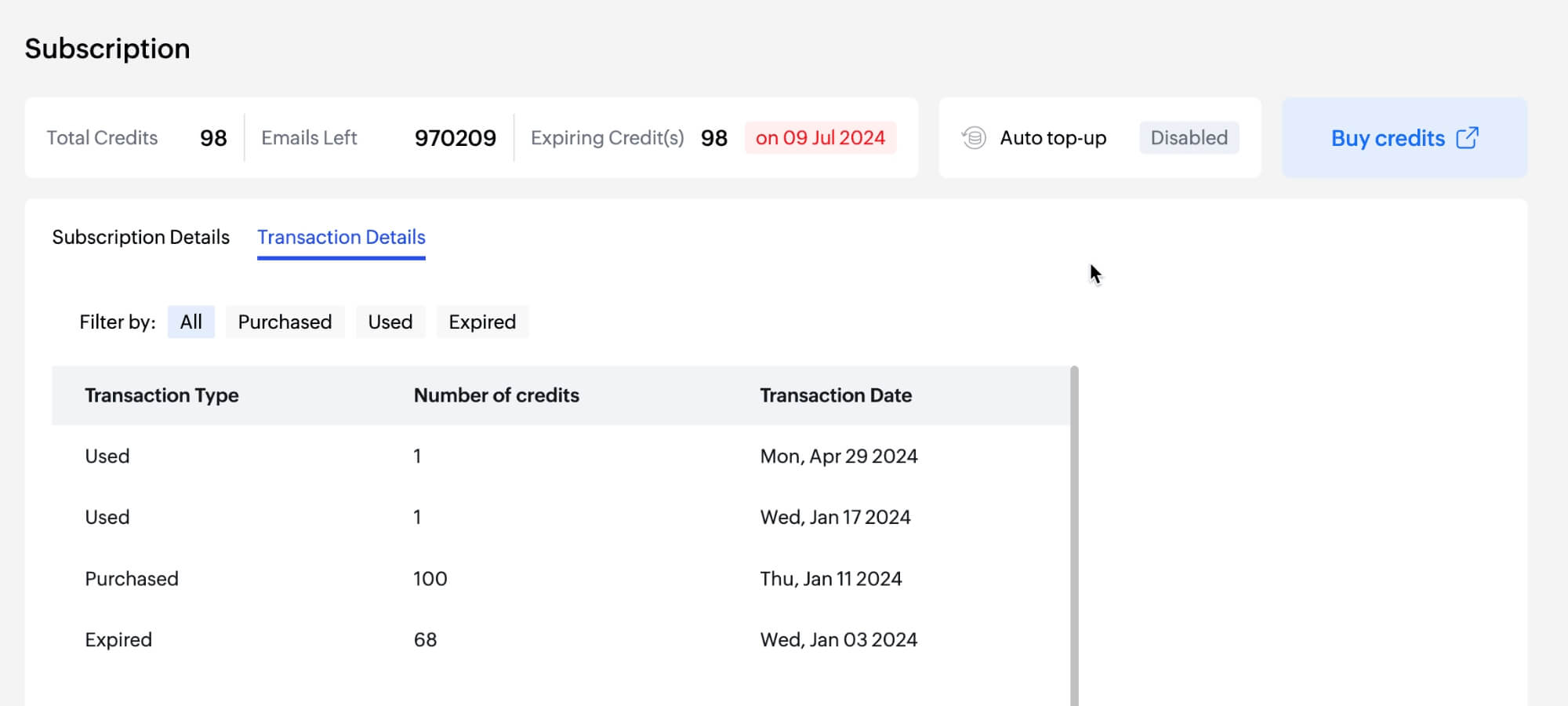
The subscription section in ZeptoMail displays your purchased credits, transaction history, remaining credits, and emails. You can also purchase credits and activate auto top-up for automatic credit acquisition. To enable auto top-up, ensure your account has been reviewed and you’ve purchased credits at least once. Learn more
Manage Users
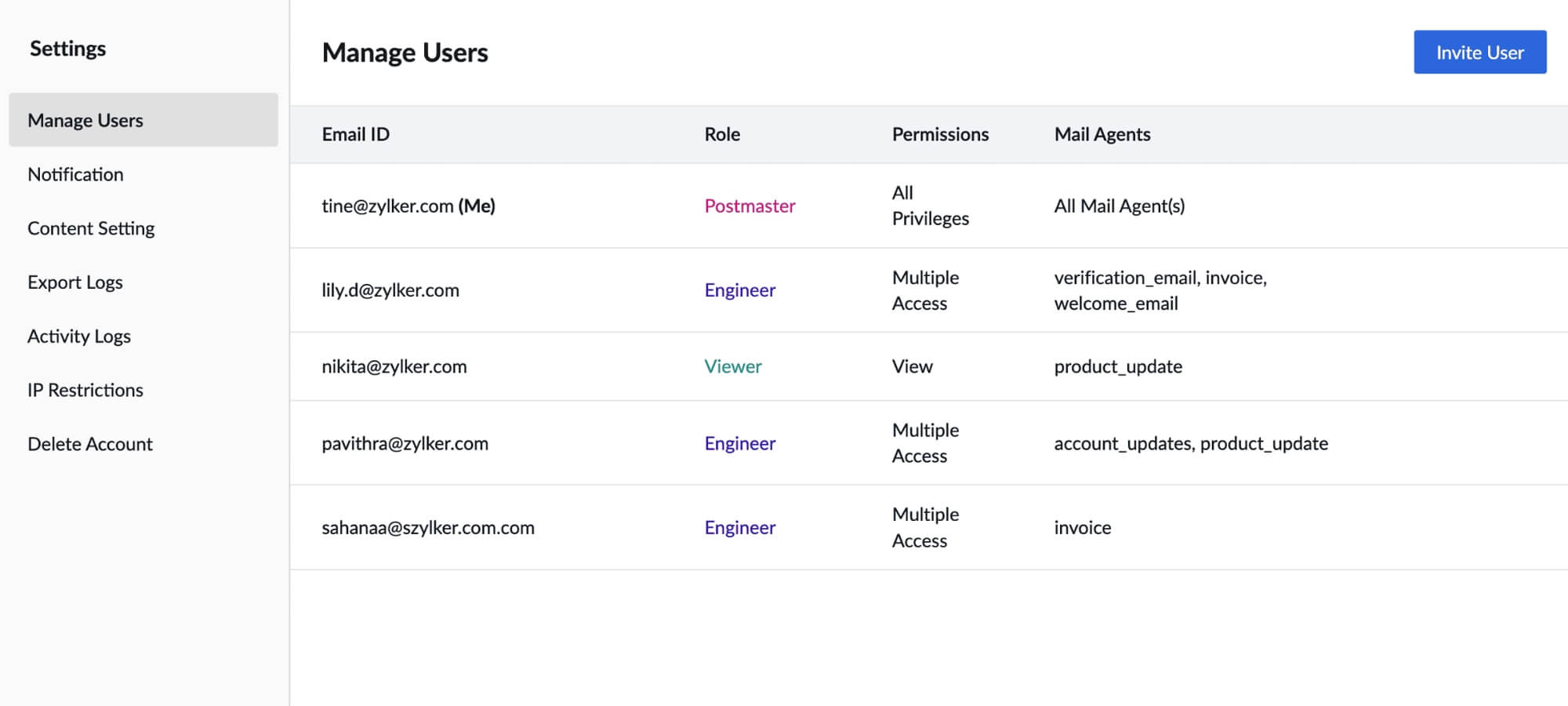
The Manage User feature allows you to manage up to 30 users within a ZeptoMail account. Each user’s role and their associated Mail Agents are displayed. ZeptoMail offers three main roles: Postmaster: (account admin with full privileges), Engineer: (multiple access), Viewer: (view-only access). Learn more
Activity Logs
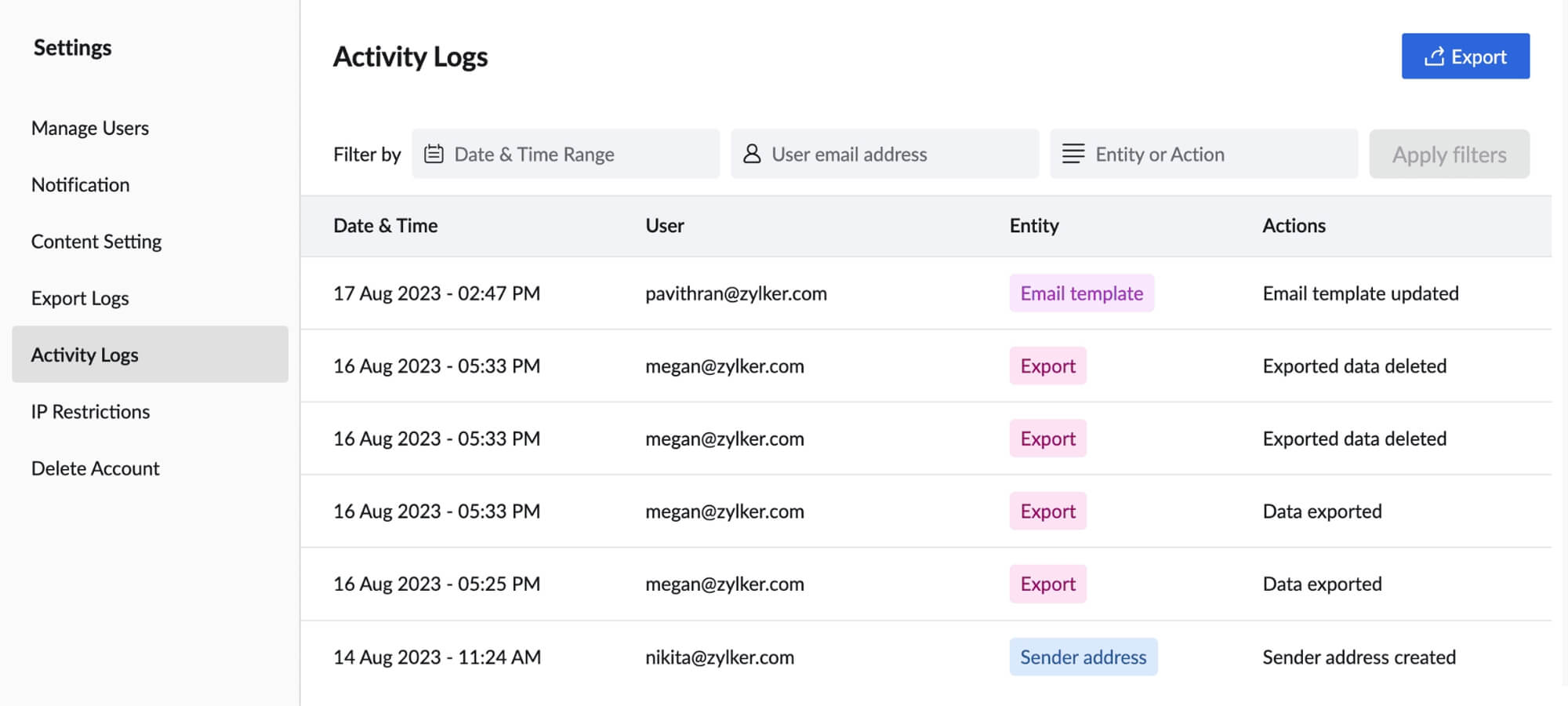
Activity Logs in ZeptoMail monitor and record user actions such as renaming files, updating Mail Agents, and creating templates. Logs can be exported for compliance purposes, with the .zip file available for three days. You can export up to 5,000 records at a time. Learn more
Content Settings

In ZeptoMail, you can store the content of your transactional emails in the Content Settings section found in the Settings menu. This saved content is accessible in the Processed Emails tab under each Mail Agent for up to 60 days. Be sure to check the “Save the email content” box to preserve your content. Learn more
IP Restrictions
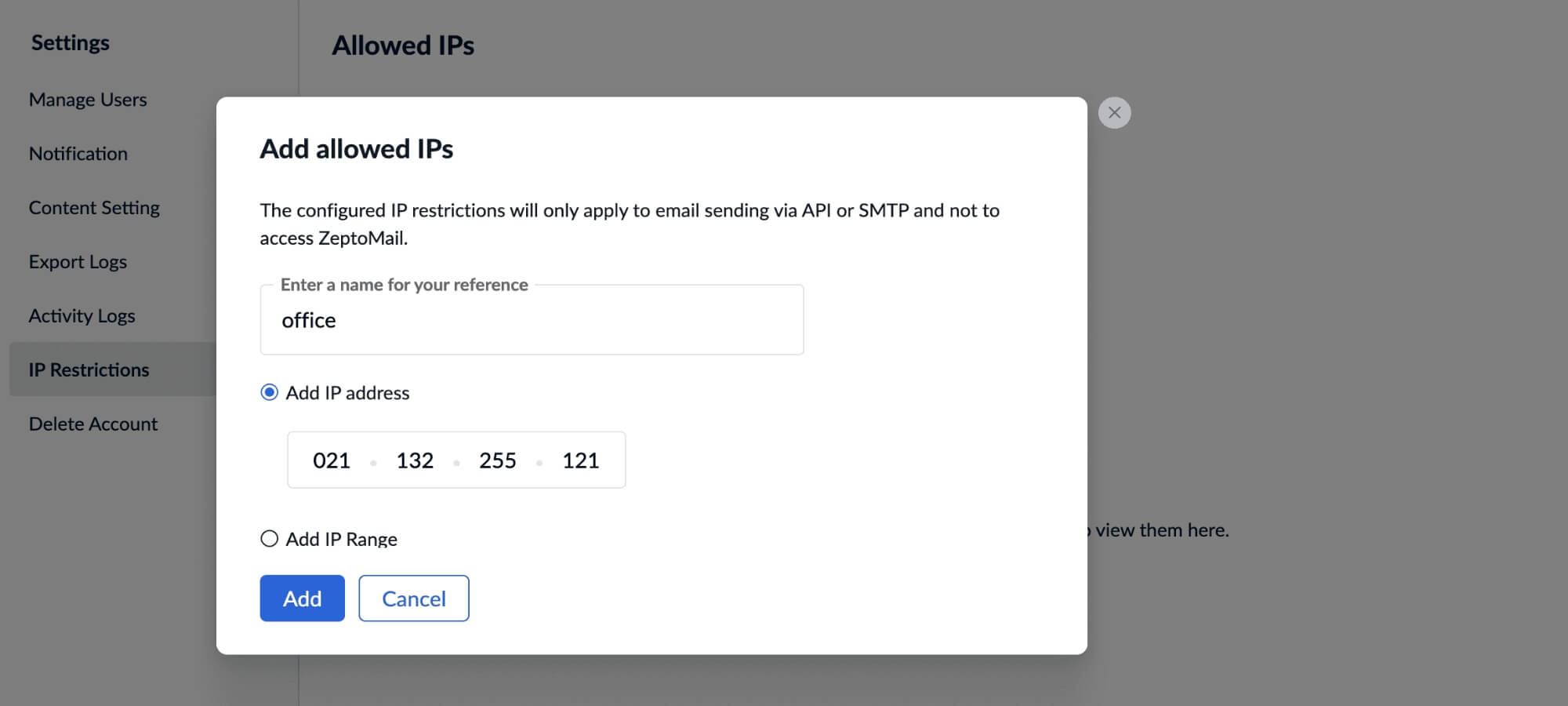
ZeptoMail’s IP restriction feature lets you create a list of authorized IPs that can send emails from your account. Adding your IPs to this list strengthens security by blocking unauthorized email sending and safeguarding against token misuse. Learn more
Export Logs
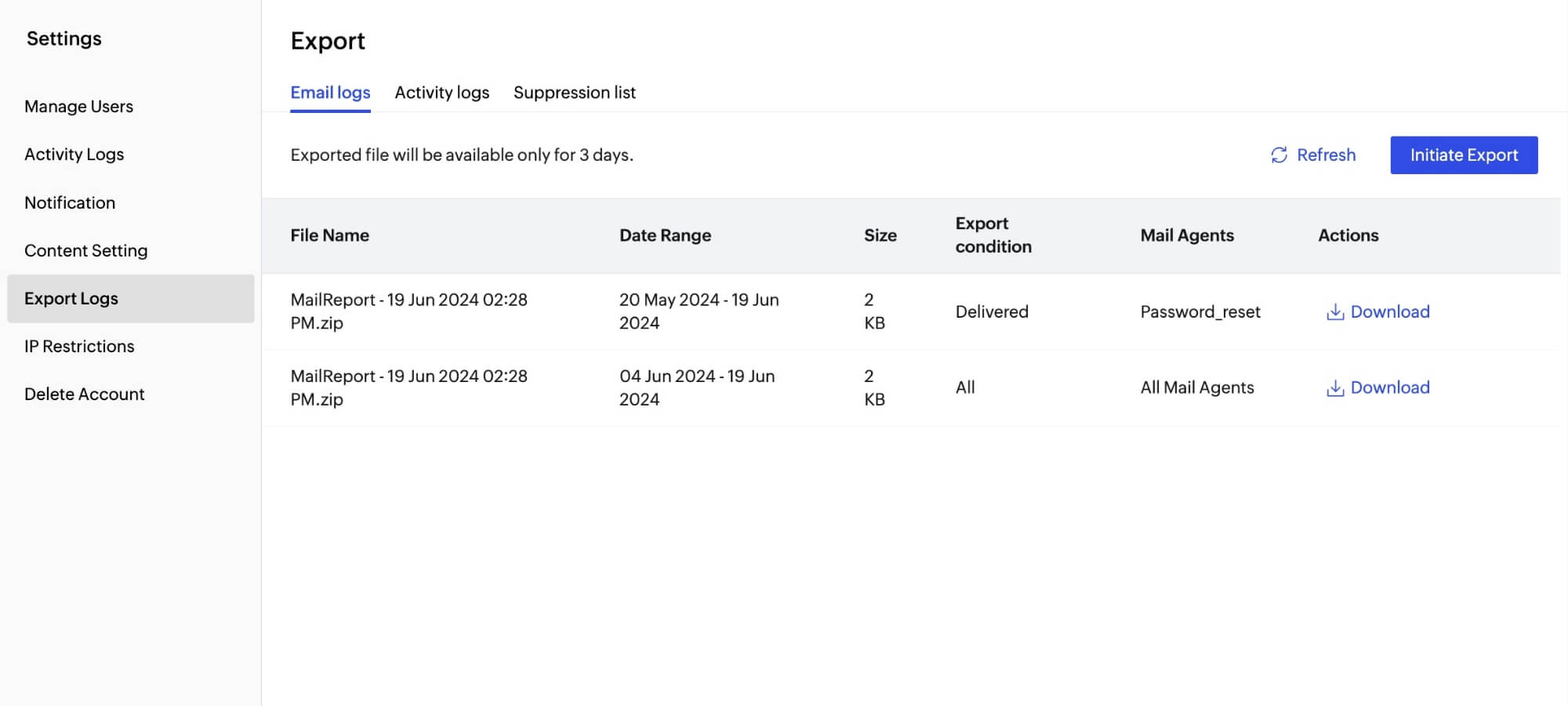
ZeptoMail lets you export email logs, activity logs, and suppression lists. These files can be securely exported with a password, ensuring your data and confidential information remain protected from unauthorized access. Learn more
Why should you choose ZeptoMail?
Exclusively Transactional
ZeptoMail provides a dedicated service that delivers emails swiftly with top-notch inbox placement.
User-friendly Interface
ZeptoMail’s interface is simple to use, ensuring seamless integration with your business.
Email Segmentation
Group your emails into Mail Agents, categorizing them by domain, application, or purpose.
No Gatekeeping
Experience all of the features without any restrictions. No updates or extra costs are needed to access ZeptoMail’s capabilities.
Comprehensive Reports
Stay informed about your activities with detailed reports and logs from ZeptoMail, where every action is meticulously recorded as data.
Flexible Pricing
Eliminate the need for monthly plan payments and unused email expenses with ZeptoMail’s pay-as-you-go pricing.
Attachment Storage
ZeptoMail's File Cache is used to store attachments for quick access during the process of sending emails.
24/7 Support
Access chat, phone, and email support around the clock for assistance with anything ZeptoMail-related.
Security and Privacy
ZeptoMail adopts a "security-first" approach in order to protect the confidentiality of transactional emails.
Frequently asked questions
How long does it take to transition to ZeptoMail?
Switching to ZeptoMail is easy and fast. Update just one line of your API code, and you can start sending transactional emails within five minutes.
Will my email deliverability be affected after transition?
No, not at all. The best thing about ZeptoMail it doesn’t compromise on email deliverability. ZeptoMail safeguards your sender reputation and ensures high deliverability by separating transactional emails from marketing emails on shared IPs. So when you start sending emails from ZeptoMail, you don’t have to worry about deliverability.
How much does it cost to transition to ZeptoMail?
There are no additional charges for transitioning. Pay only for the emails that you send using ZeptoMail.
Is ZeptoMail more affordable than Amazon SES?
While Amazon SES offers a more cost-effective solution compared to ZeptoMail, it’s important to note that it may require a higher level of technical expertise to effectively use its services. Additionally, users should be aware that there are extra charges for adding attachments to emails sent through Amazon SES.
Is it possible to start sending emails right after you create an account?
Yes. As soon as you create your account and verify your domain, you’ll be able to send up to 10,000 emails, limited to 100 emails per day. Additional credits can be purchased after your account has been reviewed.
How can you purchase more ZeptoMail credits?
You have the option to buy credits either from the dashboard panel or the subscription panel. Each ZeptoMail credit allows you to send 10,000 emails and remains valid for 6 months.
Why do you need a Mail Agent?
A Mail Agent is used to group all of your transactional emails. You’ll be sending different transactional emails like OTPs, password rests, and payment confirmations from ZeptoMail. So, ZeptoMail uses Mail Agents to manage them efficiently.
What happens if you run out of email credits?
You will no longer be able to send emails. To avoid this, turn on the auto top-up in your account to automatically purchase email credits when you reach your predefined limit.