Serverless Architecture - An Overview
Serverless architecture provides a platform for developers to execute their own codes in response of various business events. These codes can be run without provisioning or managing servers. These codes once written can be triggered from any other third party services or it can be called directly triggered from any web or mobile app.
Although called “Serverless”, these codes require servers to run them, but the developers or organizations need not buy, provision, or rent any servers to run their code.
The serverless computing framework is used to build functions to execute certain business logics. You can write the functions in scripting languages like Deluge and Java, and Zoho CRM takes care of running them.
How Functions REST API Work?
Highlights
Write functions in Deluge and Java.
Easy to code and deploy - runs in Zoho CRM.
Portable - Trigger the function from any source. You can have the functions as API endpoints, so that you can trigger them from any user-defined action performed.
Event-driven and easily scalable instantly.
See also:
Integration tasks for Functions using the Version 2.0 of APIs.
Set up Connections - Connect your Zoho CRM with any third-party application by invoking their APIs in a function.
Availability
Profile Permission Required: Admins and Users with "Modules Customization" and "Manage Workflow" permission can access this feature.

Dashboard
The dashboard for the serverless architecture lets you keep track of all the functions that executed or failed, and helps you view the usage statistics of all your functions. Learn more.
Gallery
A pre-defined list of deluge functions are available in your CRM, by default. They involve the most common and preferred actions that are to be performed on data stored in your CRM. For instance, creating deal records automatically for periodically recurring deals. Learn more.
Failures
A function execution fails due to reasons such as an error in the logic, error in the data passed to the function etc. The Failures tab lets you keep track of the functions that failed, reason for failure, and the option to rerun them. Learn more.
Deployment
You can use the editor available in Zoho CRM to write your script either in Deluge or Java.
Go to Setup > Developer Space > Functions.
The below image shows where you select your preferred language and take a look at how the configured functions work.
Click the +New Function button to create a function.
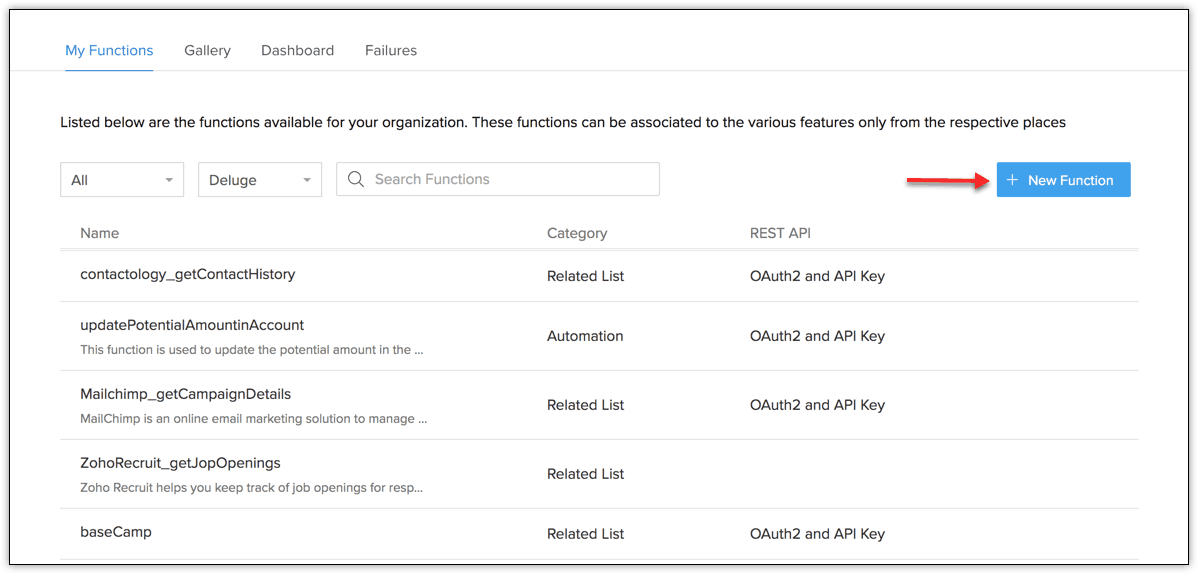
Functions - Examples
You can write the function script in the editor available inside Zoho CRM or you can upload the script as a zip file.
Apart from Deluge, you can now write your functions in Java.
Deluge Functions
Here's an example to create a record in the Leads module using the Deluge script.
data = Map(); data.putAll({"Last_Name":"Bruce Wills", "Company":"Zillum"}); optionalMap = Map(); optionalMap.put("trigger", ["workflow","approval", "blueprint"]); // pass "trigger" as empty [] to not execute workflow resp = zoho.crm.create("Leads", data, optionalMap);
Java Functions
Here's an example to create a record in the Leads module using a function written in Java.
// Don't remove below imports. import com.zoho.cloud.function.Context; import com.zoho.cloud.function.basic.*; import org.json.simple.JSONObject; import org.json.simple.JSONArray; import com.zoho.cloud.task.crm.CRM; import org.json.simple.parser.JSONParser; /* - The Class which implements ZCFunction will act as the main of the JAVA Package - Java Execution starts from the method name 'runner'. */ public class createRecord implements ZCFunction { public void runner(Context context, BasicIO basicIO) throws Exception { String request = (String) basicIO.getParameter("crmAPIRequest"); JSONParser parser = new JSONParser(); JSONObject requestObj = (JSONObject) parser.parse(request); String last_Name = (String) requestObj.get("Last_Name"); String first_Name = (String) requestObj.get("First_Name"); String company = (String) requestObj.get("Company"); String mobile = (String) requestObj.get("Mobile"); CRM crm = (CRM) context.getIntegrationTask().getService("CRM"); JSONObject data = new JSONObject(); JSONArray arr = new JSONArray(); JSONObject leadInfo = new JSONObject(); leadInfo.put("Last_Name", last_Name); leadInfo.put("First_Name", first_Name); leadInfo.put("Company", company); leadInfo.put("Mobile", mobile); arr.add(leadInfo); data.put("data", arr); String response = crm.getApi("v2").createRecord("Leads", data); basicIO.write(response); } }