Multi-User and Multi-DC Support
In the PHP SDK, multi-user functionality is achieved using the switchUser() method. To use this method, you need to provide the user, environment, token, and SDK configuration details.
Please note that only one user can send requests at a time. If another user need to send requests, the switchUser() method must be used prior to sending the requests.
(new InitializeBuilder())
->user($user)
->environment($environment)
->token($token)
->SDKConfig($configInstance)
->switchUser();
To Remove a user's configuration in SDK. Use the below code
Initializer::removeUserConfiguration($user, $environment);
<?php
namespace multiuser;
use com\zoho\api\authenticator\OAuthBuilder;
use com\zoho\api\authenticator\store\DBBuilder;
use com\zoho\api\authenticator\store\FileStore;
use com\zoho\crm\api\InitializeBuilder;
use com\zoho\crm\api\UserSignature;
use com\zoho\crm\api\dc\USDataCenter;
use com\zoho\crm\api\dc\EUDataCenter;
use com\zoho\api\logger\LogBuilder;
use com\zoho\api\logger\Levels;
use com\zoho\crm\api\SDKConfigBuilder;
use com\zoho\crm\api\ProxyBuilder;
use com\zoho\crm\api\Initializer;
use com\zoho\crm\api\record\RecordOperations;
use com\zoho\crm\api\record\GetRecordsHeader;
use com\zoho\crm\api\HeaderMap;
use com\zoho\crm\api\ParameterMap;
require_once 'vendor/autoload.php';
class MultiThread
{
public function main()
{
$logger = (new LogBuilder())
->level(Levels::INFO)
->filePath("/Users/user_name/Documents/php_sdk_log.log")
->build();
$environment1 = USDataCenter::PRODUCTION();
$user1 = new UserSignature("abc1@zoho.com");
$tokenstore = (new DBBuilder())->host("hostName")->databaseName("dataBaseName")->userName("userName")->password("password")->portNumber("portNumber")->tableName("tableName")->build();
$token1 = (new OAuthBuilder())
->clientId("clientId")
->clientSecret("clientSecret")
->grantToken("grantToken")
->redirectURL("redirectURL")
->build();
$autoRefreshFields = false;
$pickListValidation = false;
$connectionTimeout = 2;
$timeout = 2;
$sdkConfig = (new SDKConfigBuilder())->autoRefreshFields($autoRefreshFields)->pickListValidation($pickListValidation)->sslVerification($enableSSLVerification)->connectionTimeout($connectionTimeout)->timeout($timeout)->build();
$resourcePath ="/Users/user_name/Documents/phpsdk-application";
(new InitializeBuilder())
->user($user1)
->environment($environment1)
->token($token1)
->store($tokenstore)
->SDKConfig($configInstance)
->resourcePath($resourcePath)
->logger($logger)
->initialize();
$this->getRecords("Leads");
$environment2 = EUDataCenter::PRODUCTION();
$user2 = new UserSignature("abc2@zoho.eu");
$token2 = (new OAuthBuilder())
->clientId("clientId2")
->clientSecret("clientSecret2")
->refreshToken("refreshToken2")
->redirectURL("redirectURL2")
->build();
$this->getRecords("Leads");
// Initializer::removeUserConfiguration($user1, $environment1);
(new InitializeBuilder())
->user($user2)
->environment($environment2)
->token($token2)
->SDKConfig($configInstance)
->switchUser();
$this->getRecords("Contacts");
}
public function getRecords($moduleAPIName)
{
try
{
$recordOperations = new RecordOperations();
$paramInstance = new ParameterMap();
$paramInstance->add(GetRecordsParam::approved(), "false");
$headerInstance = new HeaderMap();
$ifmodifiedsince = date_create("2020-06-02T11:03:06+05:30")->setTimezone(new \DateTimeZone(date_default_timezone_get()));
$headerInstance->add(GetRecordsHeader::IfModifiedSince(), $ifmodifiedsince);
//Call getRecord method that takes paramInstance, moduleAPIName as parameter
$response = $recordOperations->getRecords($moduleAPIName,$paramInstance, $headerInstance);
echo($response->getStatusCode() . "\n");
print_r($response->getObject());
echo("\n");
}
catch (\Exception $e)
{
print_r($e);
}
}
}
$obj = new MultiThread();
$obj->main();
?>
The program execution starts from main().
The details of user1 is given in the variables user1, token1, environment1.
Similarly, the details of another user user2 is given in the variables user2, token2, environment2.
Note:If you have enabled multi-dc support in the client app, the token object will be DC specific. In multi-DC enabled case, the client-id will be the same for different DCs for the same org, but the tokens (grant, refresh and access) will be different. The client-secret could be the same or different, depending on how you have configured it.
Then, the Switch user function is used to switch between the User 1 and User 2 as required.
Based on the latest switched user, the $this->getRecords($moduleAPIName) will fetch record.
SDK Sample Code
<?php
namespace index;
use com\zoho\api\authenticator\OAuthToken;
use com\zoho\api\authenticator\store\DBStore;
use com\zoho\api\authenticator\store\FileStore;
use com\zoho\crm\api\Initializer;
use com\zoho\crm\api\UserSignature;
use com\zoho\crm\api\SDKConfigBuilder;
use com\zoho\crm\api\dc\USDataCenter;
use com\zoho\api\logger\Logger;
use com\zoho\api\logger\Levels;
use com\zoho\crm\api\record\RecordOperations;
use com\zoho\crm\api\HeaderMap;
use com\zoho\crm\api\ParameterMap;
use com\zoho\crm\api\record\GetRecordsHeader;
use com\zoho\crm\api\record\GetRecordsParam;
use com\zoho\crm\api\record\ResponseWrapper;
require_once 'vendor/autoload.php';
class Record
{
public static function getRecord()
{
/*
* Create an instance of Logger Class that requires the following
* level -> Level of the log messages to be logged. Can be configured by typing Levels "::" and choose any level from the list displayed.
* filePath -> Absolute file path, where messages need to be logged.
*/
$logger = (new LogBuilder())
->level(Levels::INFO)
->filePath("/Users/user_name/Documents/php_sdk_log.log")
->build();
//Create an UserSignature instance that takes user Email as parameter
$user = new UserSignature("abc@zoho.com");
/*
* Configure the environment
* which is of the pattern Domain::Environment
* Available Domains: USDataCenter, EUDataCenter, INDataCenter, CNDataCenter, AUDataCenter
* Available Environments: PRODUCTION(), DEVELOPER(), SANDBOX()
*/
$environment = USDataCenter::PRODUCTION();
//Create a Token instance
$token = (new OAuthBuilder())
->clientId("clientId")
->clientSecret("clientSecret")
->refreshToken("refreshToken")
->redirectURL("redirectURL")
->build();
//$tokenstore = (new DBBuilder())->build();
$tokenstore = (new DBBuilder())->host("hostName")->databaseName("dataBaseName")->userName("userName")->password("password")->portNumber("portNumber")->tableName("tableName")->build();
// $tokenstore = new FileStore("absolute_file_path");
$autoRefreshFields = false;
$pickListValidation = false;
$connectionTimeout = 2;
$timeout = 2;
$sdkConfig = (new SDKConfigBuilder())->autoRefreshFields($autoRefreshFields)->pickListValidation($pickListValidation)->sslVerification($enableSSLVerification)->connectionTimeout($connectionTimeout)->timeout($timeout)->build();
$resourcePath ="/Users/user_name/Documents/phpsdk-application";
/*
* Set the following in InitializeBuilder
* user -> UserSignature instance
* environment -> Environment instance
* token -> Token instance
* store -> TokenStore instance
* SDKConfig -> SDKConfig instance
* resourcePath -> resourcePath -A String
* logger -> Log instance (optional)
* requestProxy -> RequestProxy instance (optional)
*/
(new InitializeBuilder())
->user($user)
->environment($environment)
->token($token)
->store($tokenstore)
->SDKConfig($configInstance)
->resourcePath($resourcePath)
->logger($logger)
->requestProxy($requestProxy)
->initialize();
try
{
$recordOperations = new RecordOperations();
$paramInstance = new ParameterMap();
$paramInstance->add(GetRecordsParam::approved(), "both");
$headerInstance = new HeaderMap();
$ifmodifiedsince = date_create("2020-06-02T11:03:06+05:30")->setTimezone(new \DateTimeZone(date_default_timezone_get()));
$headerInstance->add(GetRecordsHeader::IfModifiedSince(), $ifmodifiedsince);
$moduleAPIName = "Leads";
//Call getRecord method that takes paramInstance, moduleAPIName as parameter
$response = $recordOperations->getRecords($moduleAPIName,$paramInstance, $headerInstance);
if($response != null)
{
//Get the status code from response
echo("Status Code: " . $response->getStatusCode() . "\n");
//Get object from response
$responseHandler = $response->getObject();
if($responseHandler instanceof ResponseWrapper)
{
//Get the received ResponseWrapper instance
$responseWrapper = $responseHandler;
//Get the list of obtained Record instances
$records = $responseWrapper->getData();
if($records != null)
{
$recordClass = 'com\zoho\crm\api\record\Record';
foreach($records as $record)
{
//Get the ID of each Record
echo("Record ID: " . $record->getId() . "\n");
//Get the createdBy User instance of each Record
$createdBy = $record->getCreatedBy();
//Check if createdBy is not null
if($createdBy != null)
{
//Get the ID of the createdBy User
echo("Record Created By User-ID: " . $createdBy->getId() . "\n");
//Get the name of the createdBy User
echo("Record Created By User-Name: " . $createdBy->getName() . "\n");
//Get the Email of the createdBy User
echo("Record Created By User-Email: " . $createdBy->getEmail() . "\n");
}
//Get the CreatedTime of each Record
echo("Record CreatedTime: ");
print_r($record->getCreatedTime());
echo("\n");
//Get the modifiedBy User instance of each Record
$modifiedBy = $record->getModifiedBy();
//Check if modifiedBy is not null
if($modifiedBy != null)
{
//Get the ID of the modifiedBy User
echo("Record Modified By User-ID: " . $modifiedBy->getId() . "\n");
//Get the name of the modifiedBy User
echo("Record Modified By User-Name: " . $modifiedBy->getName() . "\n");
//Get the Email of the modifiedBy User
echo("Record Modified By User-Email: " . $modifiedBy->getEmail() . "\n");
}
//Get the ModifiedTime of each Record
echo("Record ModifiedTime: ");
print_r($record->getModifiedTime());
print_r("\n");
//Get the list of Tag instance each Record
$tags = $record->getTag();
//Check if tags is not null
if($tags != null)
{
foreach($tags as $tag)
{
//Get the Name of each Tag
echo("Record Tag Name: " . $tag->getName() . "\n");
//Get the Id of each Tag
echo("Record Tag ID: " . $tag->getId() . "\n");
}
}
//To get particular field value
echo("Record Field Value: " . $record->getKeyValue("Last_Name") . "\n");// FieldApiName
echo("Record KeyValues : \n" );
//Get the KeyValue map
foreach($record->getKeyValues() as $keyName => $value)
{
echo("Field APIName" . $keyName . " \tValue : ");
print_r($value);
echo("\n");
}
}
}
}
}
}
catch (\Exception $e)
{
print_r($e);
}
}
}
Record::getRecord();
?>
Record Response
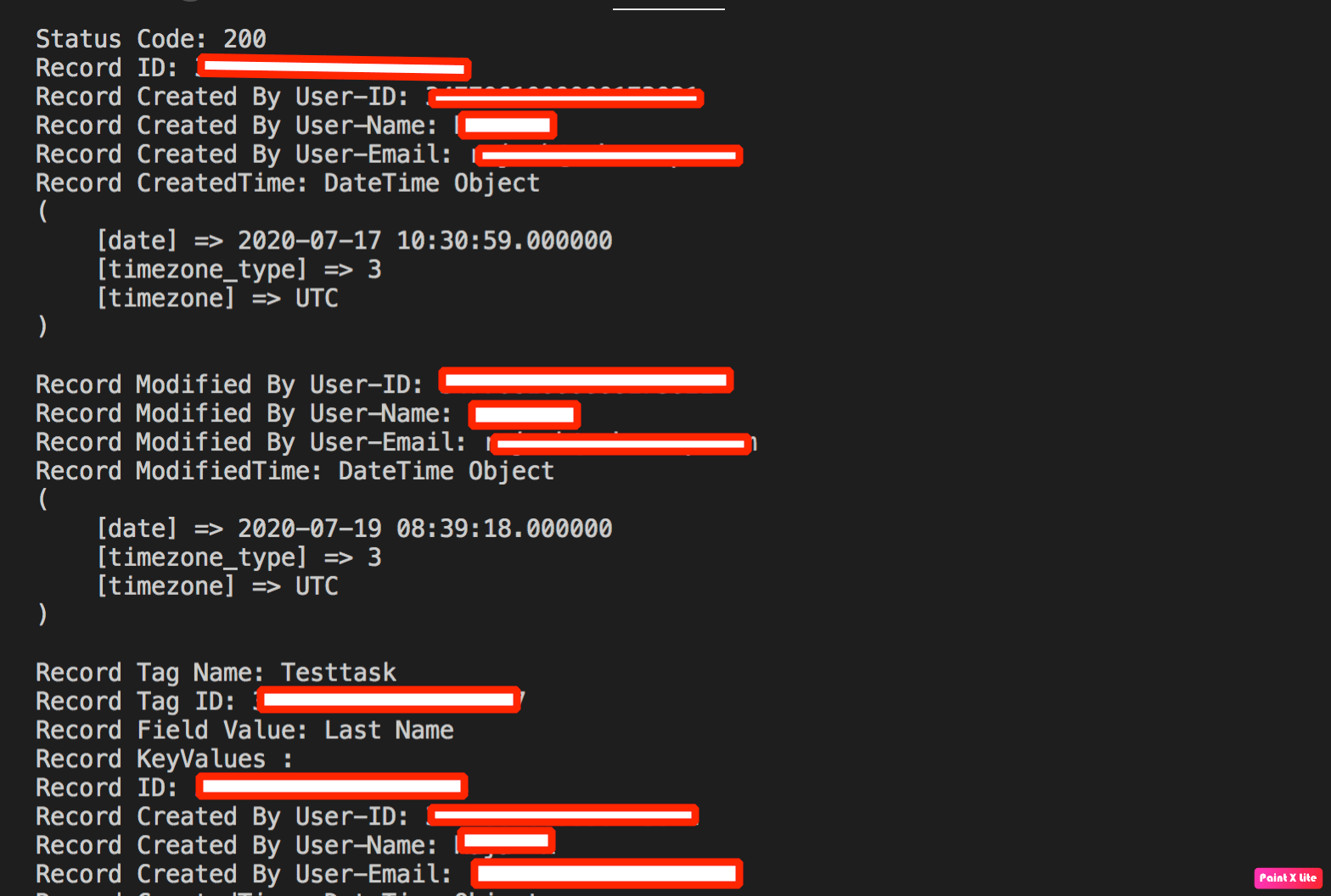